While loop in R
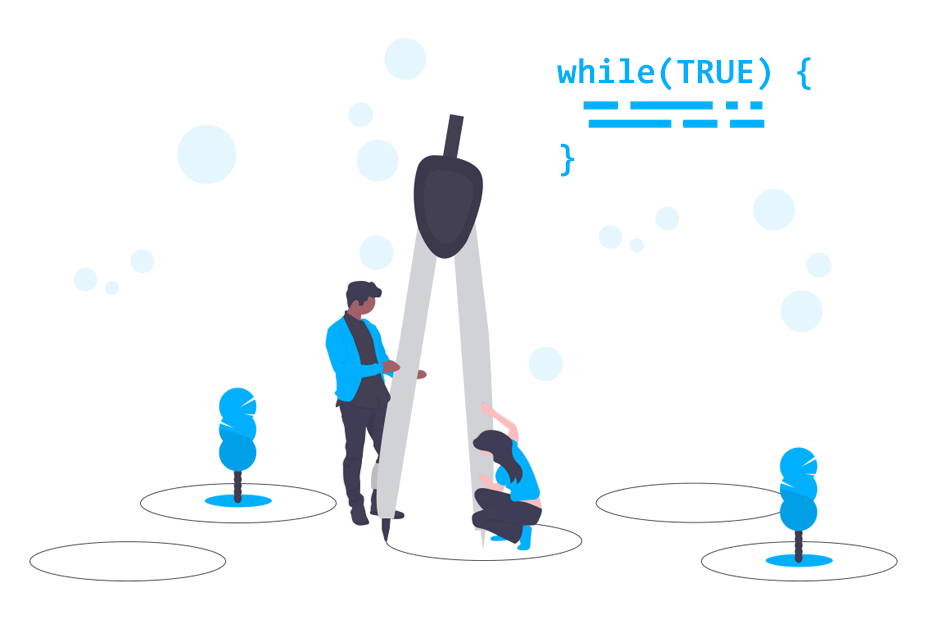
A while loop in R programming is a function designed to execute some code until a condition is met. While the logical condition is TRUE, the code won’t stop executing. This type of loop is very useful for simulation studies. The R while loop is very similar to the for loop, but in the second you will define the number of iterations to execute.
While loop syntax
The while loop will execute some code until a logical condition is met. Therefore, while some condition is TRUE, R will DO something. For a while loop you need to use the while
function with the following syntax:
while (logic_condition) {
# Code
}
Take care! While loops may never stop if the logic condition is always TRUE.
Examples of while loop in R
In this section we are going to show you some use cases for better understanding of the R while loop.
Factorial in R using while loop
The factorial of a non-negative integer is the multiplication of the integers from that number to 1. In mathematics is denoted by !
. For example, the factorial of 3 is 3! = 3 \(\cdot\) 2 \(\cdot\) 1 = 6. Note that the factorial of 0 is 1.
factorialR <- function(x) {
if (x == 0) {
res <- 1
} else {
res <- x
while(x > 1){
res <- (x - 1) * res
x <- x - 1
}
}
return(res)
}
factorialR(8)
factorialR(0)
40320
1
However, it should be mentioned that these results could be achieved in different ways. In fact, in R there is already a base function named factorial
, which is more efficient than the one in our example, as it is written in C.
First square exceeding some number with while loop
Suppose we want to know the first positive integer number which square exceeds 4000, we can do:
# Variable initialization
n <- 0
square <- 0
# While loop
while(square <= 4000) {
n <- n + 1
square <- n ^ 2
}
# Results
n # 64
square # 4096
Sum of two vectors
As a last example, we can create a while loop that sums two vectors. In this case we are going to sum the vectors named x
and y
.
x <- c(1, 2, 3, 4)
y <- c(0, 0, 5, 1)
n <- length(x)
i <- 0
z <- numeric(n)
while (i <= n) {
z[i] <- x[i] + y[i]
i <- i + 1
}
z
1 2 8 5
Obviously this could be achieved just with the +
operator.
Simulation of n random trails inside a circle
Suppose you want to simulate n random trails inside a circle of radius r: For that purpose you can do the following:
n <- 5 # Number of trails
radius <- 1 # Circle radius
counter <- 0
data <- matrix(nrow = n, ncol = 2)
while (counter < n) {
xaux <- runif(2, min = -radius, max = radius)
if (sqrt(xaux[1] ** 2 + xaux[2] ** 2) < radius) {
counter <- counter + 1
data[counter, ] <- xaux
}
}
data
[, 1] [, 2]
[1,] 0.7228765 -0.3418237
[2,] -0.1762560 -0.9262462
[3,] 0.7169295 0.2026573
[4,] -0.3739910 0.2346970
[5,] 0.4458186 -0.6395707