Working directory in R
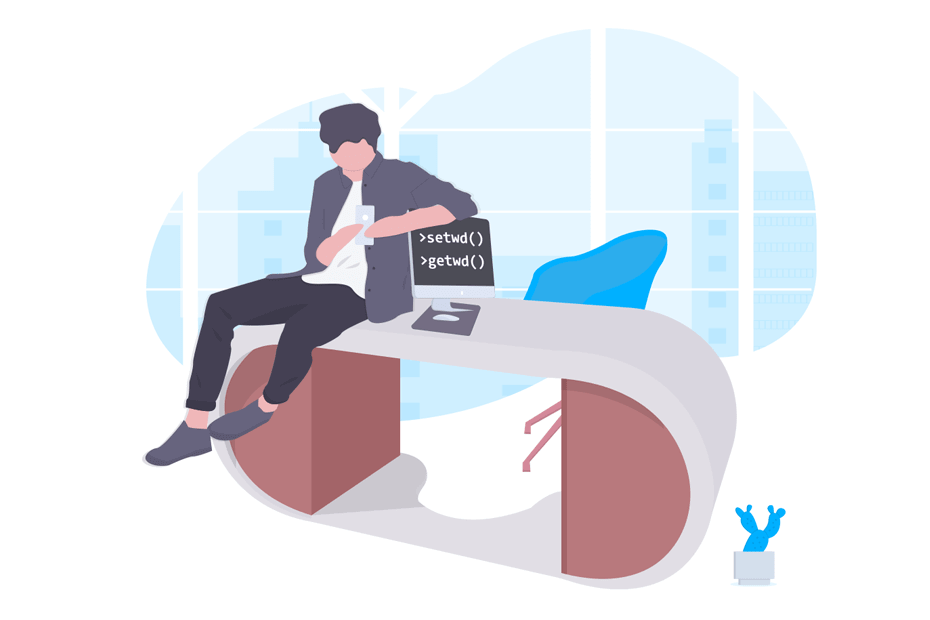
The working directory in R is the folder where you are working. Hence, it’s the place (the environment) where you have to store your files of your project in order to load them or where your R objects will be saved.
Get working directory
Getwd function
In case you want to check the directory of your R session, the function getwd
will print the current working directory path as a string. Hence, the output is the folder where all your files will be saved.
# Find the path of your working directory
getwd()
Set working directory
Setwd function
If you are wondering how to change the working directory in R you just need to call the setwd
function, specifying as argument the path of the new working directory folder.
# Set the path of your working directory
setwd("My\\Path")
setwd("My/Path") # Equivalent
In case you encountered the error: ‘unexpected input in setwd’, make sure to use ‘\\’ or ‘/’ instead of ‘\’ when writing your directory path.
There are options if you don’t want to change the slash manually:
On the one hand, you could make use of the back2ForwardSlash
function of the sos
package as follows.
# install.packages(sos)
library(sos)
x <- back2ForwardSlash()
# (Enter or paste the path)
setwd(x)
On the other hand, since R 4.0.0 you can type:
setwd(r"(My\Path)")
Change working directory in RStudio
In order to create a new RStudio project go to Session → Set Working Directory and select the option you prefer. You can set to the project directory, source file location, files pane location or set a custom path.
Error: Cannot change working directory
There are several reasons that doesn’t allow to change the working directory.
- Check you didn’t misspelled the path.
- Make sure your path doesn’t contain invalid characters, as accents.
- Make sure you have admin permissions.
- Use the double backslash or single slash.
Create an RStudio project
RStudio projects are very useful to organize our scripts in folders. Thus, when opening a project it will contain all the files corresponding to it. Also, the project folder will be set as the working directory when you open it, so everything you save will be saved in the project folder.
Navigate to File → New Project and create a new project from a New Directory or from an Existing Directory.
If you selected the option New Directory you will have to select New Project and then write a project name and path.
Once done, a .Rproj
file will be created and you will be able to have a project with all your files without the need of setting a working directory each time you open R.
Create a folder inside working directory
After setting up your working directory, you can create a new folder with the dir.create
function inside the main directory. For instance, you could create a new folder, set it as new working directory and come back to the main working directory the following way:
# Save your current working directory
old_wd <- getwd()
# Create a new folder
dir.create("new_folder")
# (Do your work)
# Come back to the main directory
setwd(old_wd)
Moreover, you can create nested folders with the recursive
argument and the file.path
function. We will give a more detailed explanation of the file.path
function on its corresponding section.
# Create a new folder inside other
dir.create(file.path("folder", "child_folder"), recursive = TRUE)
Remove a folder inside working directory
In case you need to remove a folder, you can call the unlink
function. It should be noted that setting the recursive
argument to TRUE
will remove all files inside the folder.
unlink("my_folder_name", recursive = TRUE)
List files of the working directory
Once you set up your working directory, you may want to know which files are inside it. For that purpose just call the dir
or the list.files
functions as illustrated in the following example.
dir()
list.files() # Equivalent
Create a file in working directory
If you need to create a new R file inside your working directory, you can use the file.create
function and specify the name of the new file as follows:
# Creating a new R file
file.create("new_file.R")
It should be noted that this command is not commonly used, as you can press Ctrl + Shift + n
in RStudio or just create a new file manually. The main use of this is command is to create a batch of new R files when working on a large project.
Remove a file in working directory
In the same way as creating a new file, you can remove or delete a file inside your directory with the file.remove
function typing:
# Deleting the file 'new_file.R'
file.remove("new_file.R")
Get file path and info
You can also check a file path with the file.path
function and even obtain information about some file using the file.info
function.
# Creating some file
file.create("my_file.R")
# Path of some file
file.path("my_file.R")
# Info about our R file
file.info("my_file.R")
size isdir mode mtime ctime atime exe
new_file.R 0 FALSE 666 2020-03-22 16:02:54 2020-03-22 16:02:54 2020-03-22 16:02:54 no
Copy files of your working directory
If needed, you can also copy and rename an R file in your directory. For that purpose, use the file.copy
function. As an example, you can copy the file named ‘my_file.R’ and rename the copy as ‘my_copied_file.R’.
file.copy("my_file.R", "my_copied_file.R")