Read TXT in R
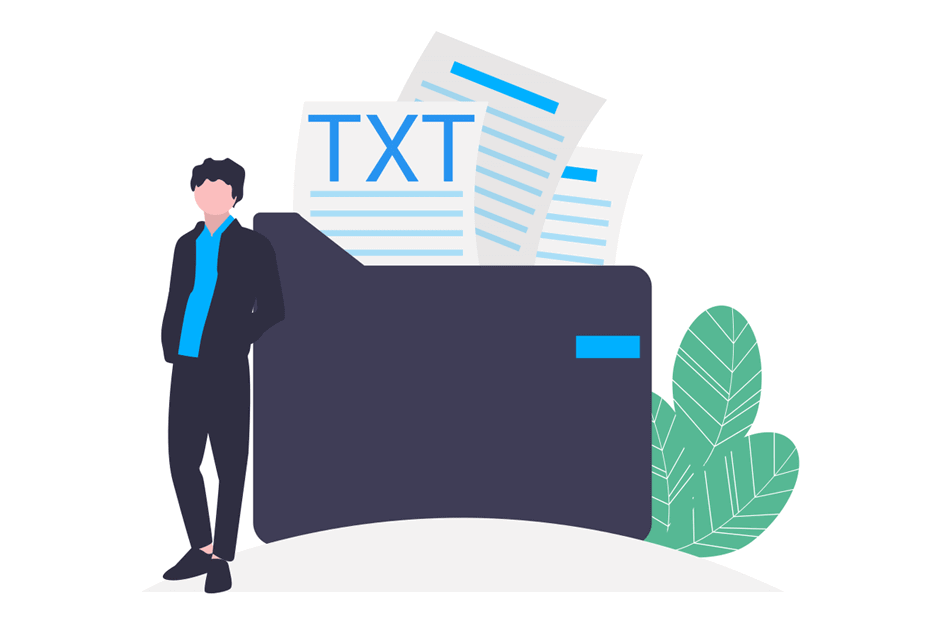
R programming language can load TXT files. If you are wondering how to read TXT files in R, the most basic function you can use is the read.table
function. In this tutorial we show you how to read TXT file in R with several examples of specific cases, as well as how to download or import TXT files from the web to work with.
How to read TXT files in R?
You can read a TXT file in R with the read.table
function. Importing TXT into R rarely needs more arguments than specified. In the following subsections we will explain two more (skip
and skipNul
) if needed, but in case you want to know all the arguments you can find them in the read.table function documentation or calling ?read.table
. This basic syntax affects to almost all TXT data files.
read.table(file, # TXT data file indicated as string or full path to the file
header = FALSE, # Whether to display the header (TRUE) or not (FALSE)
sep = "", # Separator of the columns of the file
dec = ".") # Character used to separate decimals of the numbers in the file
Consider, for instance, that yo have a TXT file called my_file.txt
and you have put it in your R working directory. You can read it with the following code if you want to also display the header (column names).
data <- read.table(file = "my_file.txt", header = TRUE)
head(data)
The output of a TXT file read with read.table
function will be of class “data.frame”.
In case you have the file in other directory than your working directory, you will need to specify the full path where the data file is.
data <- read.table(file = "C:\My_path\my_file.txt", header = TRUE)
data <- read.table(file = "C:/My_path/my_file.txt", header = TRUE) # Equivalent
There also two functions (read.delim
and read.delim2
) to deal with delimited files by default. This functions have the following default arguments:
read.delim(file = "my_file.txt" header = TRUE, sep = "t", dec = ".")
read.delim2(file = "my_file.txt", header = TRUE, sep = "t", dec = ",")
Function | header | sep | dec |
---|---|---|---|
read.table | FALSE | “” | “.” |
read.delim | TRUE | “\t” | “.” |
read.delim2 | TRUE | “\t” | “,” |
Skip rows of a TXT file
Sometimes the TXT files you are reading contain some lines of text before the dataset. To solve this issue you can use the skip
argument, that defaults to 0. Consider, for instance, that there are 5 lines of text before your data, so you can read the file the following way:
# Skip 5 rows
read.table(file = "my_file.txt", skip = 5)
How to identify NULL values in a TXT file?
If your TXT data contains NULL
values, you can set the skipnul
argument to TRUE
to skip them.
read.table(file = "C:\My_path\my_file", skipnul = TRUE)
Import TXT from URL
In case you have a TXT file hosted in some website, you can open it without downloading it. You just need to pass the URL as string to the first argument.
url <- "http://courses.washington.edu/b517/Datasets/string.txt"
data <- read.table(url, header = TRUE)
head(data)
Download TXT file in R
Now that you know how to read a TXT in R, it should be noticed that you can directly download a TXT file in R to your working directory with the download.file
function, passing the link as the first argument and the name you want to put to the .txt file as the second.
getwd() # Path where the file will be downloaded
url <- "http://courses.washington.edu/b517/Datasets/string.txt"
download.file(url, "my_file.txt")
If you don’t want to download the file to the working directory, you can specify the path where you want the file to be downloaded.
download.file(url, "C:\folder\my_file.txt")