The repeat loop in R
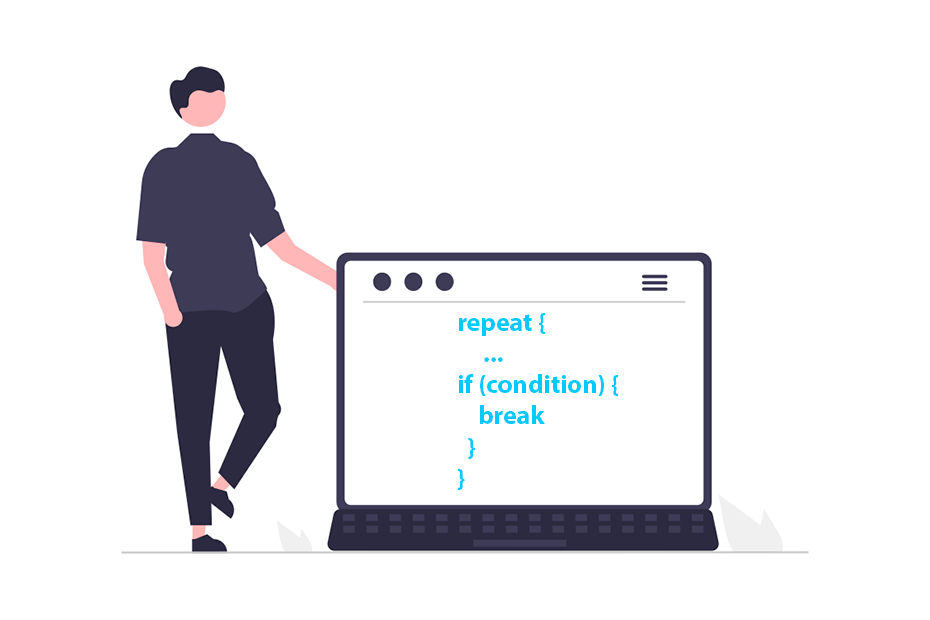
The repeat
loop is a general-purpose loop in R that iterates a block of code or process indefinitely until a specific condition is met or until the loop is explicitly terminated using the break
statement. It is similar to, but differs from, a for
loop, which iterates over a predefined sequence.
It is important to note that, in many cases, the for loop remains the clearest and most concise option for iteration over predefined sequences. The use of the repeat
loop is generally reserved for situations where the control flow logic is more complex or where more flexibility is needed in determining the output condition.
Syntax of the repeat
statement
The syntax of the repeat
loop is the following:
repeat {
# Code to be repeated
if (condition) {
break # Terminate the loop if condition is met
}
}
The repeat
loop evaluates a process and repeats it undefined times until a break
is evaluated.
Take care! Since repeat
can create infinite loops, it’s crucial to handle them safely. Set a limit of iterations or a break condition.
Use cases of repeat loops
Infinite loops
One of the primary use cases for repeat
is to create infinite loops. For example, a simulation that runs until a certain condition is met (specially when you don’t know how many iterations are requiered to meet the condition):
i <- 1
repeat {
# Code to be repeated
print(i)
i <- i + 1
# If i > 10 the loop stops
if (i > 10) {
break
}
}
[1] 1
[1] 2
[1] 3
[1] 4
[1] 5
[1] 6
[1] 7
[1] 8
[1] 9
[1] 10
As stated before, these loops are useful when you don’t know how many iterations are needed to meet a specific condition. In the following scenario a value is divided by two until is lower than a specific threshold and using a repeat
loop can be useful or quicker if you don’t know how to calculate the number of iterations needed.
value <- 10
iter <- 1
repeat {
# Code to be repeated
value <- value / 2
# If value < 0.000000048 the loop stops
if (value < 0.000000048) {
break
}
iter <- iter + 1
}
# Print results
cat("Number of iterations:", iter, "\n")
cat("Value:", value)
Number of iterations: 28
Value: 3.72529e-08
The following is a more complex example that generates random uniform values until the sum of them is greater than 5. As values are pseudorandom, the number of iterations needed to meet the condition is inherently unpredictable and depends on the specific sequence of generated values, reflecting the stochastic nature of the simulation, so a repeat
loop will work really well in this scenario.
# Setting seed for reproducibility
set.seed(123)
iterations <- 0
total_sum <- 0
repeat {
# Generate a random number between 0 and 1
random_number <- runif(1)
# Accumulate the random numbers
total_sum <- total_sum + random_number
iterations <- iterations + 1
# Check the condition (e.g., total sum greater than 5)
if (total_sum > 5) {
break # Exit the loop if the condition is met
}
}
# Print results
cat("Number of iterations:", iterations, "\n")
cat("Total sum:", total_sum, "\n")
Number of iterations: 9
Total sum: 5.32586
If you remove or change the seed of the previous example, the number of iterations to meet the condition and the final sum of values might vary.
Menu-driven programs
The repeat
loops are also handy for menu-driven programs where the user is prompted to make choices until they decide to exit. The following example illustrates how to write a repeat loop that only exits when the user inputs a positive value:
# Repeat loop to validate an user input
repeat {
user_input <- readline("Input a positive number: ")
user_input <- as.numeric(user_input)
# If input is not NA and greater than 0 stops the loop
if (!is.na(user_input) && user_input > 0) {
cat("¡Valid number!\n")
break
} else {
cat("No valid number. Try again.\n")
}
}
Input a positive number: -5
No valid number. Try again.
Input a positive number: NA
No valid number. Try again.
Input a positive number: 4
¡Valid number!