The ifelse function in R
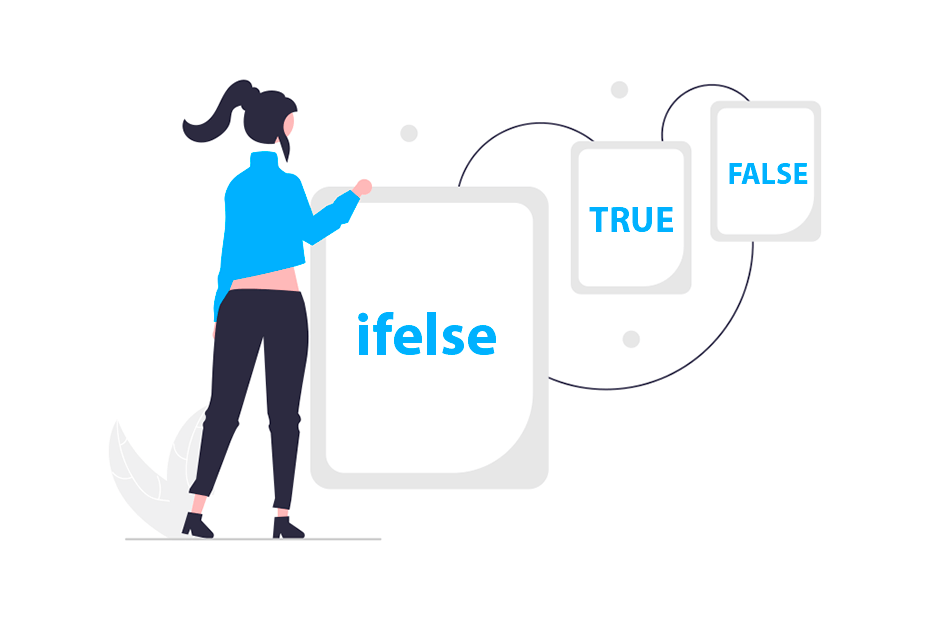
The ifelse
function is a vectorized function that allows you to apply a condition to each element of a vector and return a new vector with the results based on the evaluation of that condition. It is useful when working with vectors or columns of a dataframe and you want to apply a conditional operation element by element in an efficient way.
Syntax of the ifelse
function
The ifelse function evaluates an expression and returns the values specified in the yes
argument for elements that are TRUE
, and the values specified in the no
argument for elements that are FALSE
. The syntax of the function is the following:
ifelse(test, yes, no)
Being:
-
test
: expression to be evaluated, typically a logical comparison. -
yes
: return for theTRUE
values oftest
. -
no
: return for theFALSE
values oftest
.
The ifelse
function is used to apply conditions to each element of a vector, returning a new vector with results based on the evaluation of the condition, while if else statement is a conditional control structure that executes different blocks of code depending on the result of a single condition.
Usage and examples
Consider that you want to evaluate if a value is greater than a specific number. For that purpose, you will need to input your condition inside the test argument. The function will return the first element if the condition is TRUE
, and the second element if it is FALSE
:
# Sample value
value <- 2
# If the value is greater than 4 returns the first element or the second otherwise
res <- ifelse(value > 4, "The value is greater than 4", "The value is lower or equal to 4")
# Output
res
"The value is lower or equal to 4"
As the ifelse
function is vectorized, it is possible to apply the condition to a vector, so the output will be based on each of the elements.For instance, in the following example, the condition is applied to each of the elements of the vector, and the output is provided for each of those elements.
# Sample vector
values <- c(2, 5, 6, 1)
# For each element returns TRUE if the value is greater than 4 or FALSE otherwise
res <- ifelse(values > 4, TRUE, FALSE)
# Output
res
FALSE TRUE TRUE FALSE
ifelse
with multiple conditions
It’s important to note that you can create expressions with multiple OR (|
) or AND (&
) conditions using the ifelse
function in R. These operators allow you to build complex logical expressions to handle various conditions and make decisions based on them.
# Sample vector
x <- c("A", "C", "B")
# ifelse with several conditions
res<- ifelse(x == "C" | x == "A", "Group 1", "Group 2")
# Output
res
"Group 1" "Group 1" "Group 2"
Adding a new column to a data frame with ifelse
Given a data frame in R, you can effectively create or modify a column by applying the ifelse
function to the values within one or several columns, allowing you to evaluate a condition for each element in the specified column(s) and assign corresponding values based on whether the condition is met or not.
# Sample data frame
df <- data.frame(x = c(5, 2, 3), y = c(4, 1, 4))
# Creating a new column with ifelse
df$group <- ifelse(df$x > 3 & df$y > 2, "A", "B")
# Output
df
x y group
1 5 4 A
2 2 1 B
3 3 4 B
Nested ifelse
Nesting several ifelse
allows you to apply multiple conditional statements in a nested manner, where the result of one ifelse
function serves as the input for another ifelse
function. This enables you to create more complex decision-making structures.
x <- c(10, -1, -4)
# Nested ifelse statements
res <- ifelse(x > 0, "G1", ifelse(x > -2, "G2", "G3"))
# Output
res
"G1" "G2" "G3"
A real-life use case can be labeling grades based on scores, as shown in the following block of code:
# Sample scores
scores <- c(85, 92, 78, 65, 45, 97)
# Using ifelse to assign grades
grades <- ifelse(scores >= 90, "A",
ifelse(scores >= 80, "B",
ifelse(scores >= 70, "C",
ifelse(scores >= 60, "D", "F"))))
grades
"B" "A" "C" "D" "F" "A"
The readability and maintainability of your code may be compromised if you nest too many ifelse
statements deeply. Consider separate the logic in parts or using alternatives such as the switch function when possible.