Workspace in R
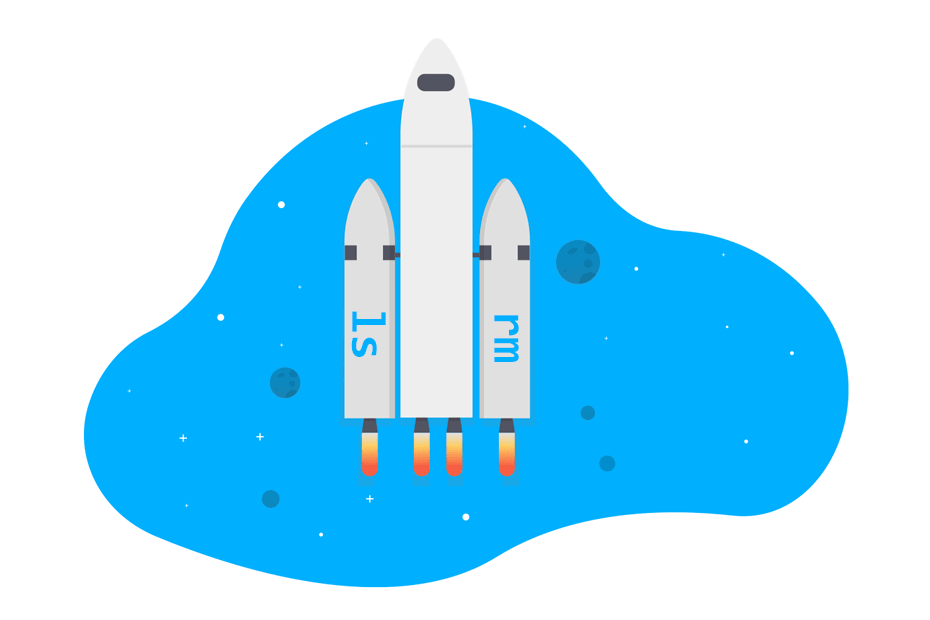
When working with R, you may want to save the data objects of your R session. This way, next time R starts, when you load that R workspace you will be able to access the objects inside it.
List workspace objects
In order to know the objects you have in memory you can use the ls
function. As an example, suppose you just have initialized your R session and you executed the following code:
a <- 1:10
b <- log(50)
Hence, when you call the ls
function the objects in memory will be displayed.
ls() # Objects in memory
"a" "b"
Clear workspace in R
Sometimes you need to clear the workspace to avoid overriding some R objects, or just to clear your session. If you are wondering how to clear the workspace in R, note that you can delete all or just some objects:
On the one hand, to clear just one object you can make use of the rm
function and specify it.
# Remove object 'a'
rm(a)
# Check if object 'a' is still in memory
a
Error: object 'a' not found
On the other, to clear the full workspace you will need to use the following code:
# Remove the full list of R objects in session
rm(list = ls())
# Check if any object is still in session
ls()
character(0)
Note that character(0)
as an output of ls function means there are no objects in workspace.
Save R workspace image
Saving the workspace in R is very easy. In case you want to save the full workspace in R, also known as workspace image (those objects that are displayed when you call the ls
function), you can use the save.image
function. The data will be saved in a file of type RData
(also known as rda
).
# Remove all the memory
rm(list = ls())
# Start coding
x <- 20
y <- 34
z <- "house"
# Save all your objects in My_Object.RData
save.image(file = "My_Object.RData")
Similar to the previous example, in case you only want to save some objects of your R workspace, you can use the sav
e function to save R data.
# Saving objects 'x' and 'y'
save(x, y, file = "My_Two_Objects.RData")
However, when you want to save only one R object it is better to use the saveRDS
function, that will save the data in the RDS format.
saveRDS(y, file = "my_object.rds")
Saving the workspace is essential when you work with scripts that take a long time to run (for example simulation studies). This way, you can load the results without the need of running the script every time you open the script.
Load workspace in R
As a consequence of saving your workspace, now you can load it so you won’t need to run the code to obtain those objects again.
On the one hand, to load the RData object you can use the load
function and call the file name.
# Loading the workspace
load("My_Two_Objects.RData")
x
20
On the other hand, to read an RDS object you can use the readRDS
function and specify the .rds
file.
readRDS("my_object.rds")
34
R command history
Related to the workspace is the code execution history. You can recover instruction lines introduced before with the top arrow of the keyboard when the focus is on the command line, in case you want to run some code again or modify something. Furthermore, you can use the history
function that shows the latest used commands.
# Show the last executed commands (25 by default)
history()
# Show the last 3 commands
history(3)