If else in R
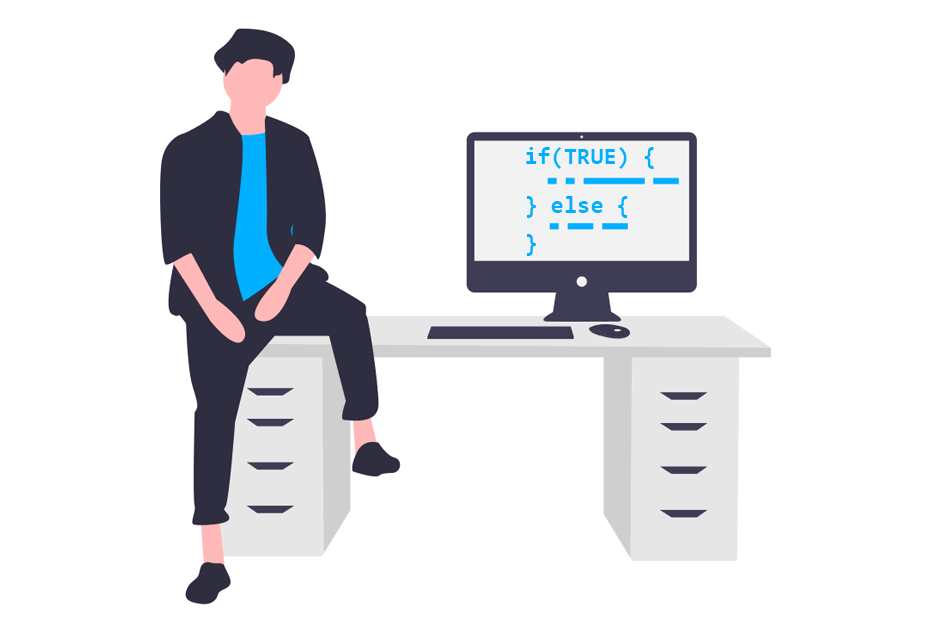
The if and else in R are conditional statements. In this tutorial we will show the syntax and some examples, with simple and nested conditions. We will also show you how to use the ifelse
function, the vectorized version of the if else condition in R.
If else statement syntax in R
The if else clause is very intuitive. You need to define one or more conditions you would like to meet to run some code, and otherwise, run other code. Hence, the condition must return TRUE
if the condition is meet or FALSE
if not.
IF some condition is meet, THEN, you run some code, ELSE, you run other code.
The R if else syntax is as follows:
if (Condition) { # The condition must return TRUE or FALSE
# Run some code
} else {
# Run other code
}
You can also write if statements in a single line without brackets, but is generally not recommended, as you could make code syntax mistakes.
if (Condition) print("Code") else print("More code")
Note you can also use the conditional statement WITHOUT the ELSE statement.
If you need several conditions to meet, you can nest if conditions (if else if …). This means you can call more if else conditions inside your previous conditions. In the following block code we show you an example with a nested if inside the first else
statement. You could nest as many ifs as you want.
if (Condition) { # The condition must return TRUE or FALSE
# Code
} else {
# Code
if(Condition 2) { # The condition must return TRUE or FALSE
# Code
} else {
# More code
}
}
Note that inside ‘Condition’ you need to use relational or boolean operators, such as AND (&), OR (|), or EQUAL (==) operators, among others.
Examples of if else in R
Now we are going to show some elaborated cases of use of if else in R. First, consider, for instance, the following function that depends on x
:
\[f(x)= \begin{cases} 0, & \text{if}\ x < 0 \\ x/10, & \text{if}\ 0 \leq x \leq 10 \\ 1, & \text{if}\ > 10 \end{cases}\]
For that purpose, you can create a function named f
and inside the function create a nested if condition to support all the possible cases.
f <- function(x) {
if (x < 0) {
0
} else {
if (x <= 10) {
x / 10
} else {
1
}
}
}
# Calling the function
f(-1) # 0
f(5) # 0.5
f(100) # 1
Second, you can define a function to check if some number is even or odd. In order to check it you can verify if the modulus of the number is equal to 0 or not, and print the corresponding result.
is_even <- function(x) {
if (x %% 2 == 0 ) {
print("x is even")
} else {
print("x is odd")
}
}
# Calling the function
is_even(5) # x is odd
is_even(10) # x is even
Ifelse statement
In R, there are lots of vectorized functions. The ifelse
function is the vectorized version of the if else statement. Say you want to call an if statement with the following structure:
if (seq(1, 5) < 5) {
print(TRUE)
} else {
print(FALSE)
}
Note that you are trying to compare a numeric vector with a unique number, so you will get the following error:
Warning message: In if (seq(1, 5) < 5) { : the condition has length > 1 and only the first element will be used.
This means the condition is comparing only the first element of the vector, as the if condition only return TRUE
or FALSE
, and not a vector of conditions. For that purpose you need to use the ifelse
function, that has the following syntax.
ifelse(vector_with_condition, value_if_TRUE, value_if_FALSE)
Now, with this function all the elements of the vector will be evaluated and the function will return a logical vector.
ifelse(seq(1, 5) < 5, TRUE, FALSE)
TRUE TRUE TRUE TRUE FALSE
You can also evaluate several conditions using the ifelse
function with nested ifelse
. Note you can nest up to 50 conditions.
ifelse(vector_with_condition,
ifelse(vector_with_condition_if_TRUE, value_if_TRUE, value_if_FALSE),
ifelse(vector_with_condition_if_FALSE, value_if_TRUE, value_if_FALSE))
R limits the ifelse to 50 nested ifelse
lines.
Ifelse examples
Suppose you want to categorize the following data of individuals as Adults or underage male/females:
x <- c("male", "male", "female", "male", "female")
y <- c(10, 14, 80, 56, 27)
Note that in the previous example the character vector x
represents if the individual is a male or a female and the numeric vector y
represents the age of the corresponding individual.
ifelse (x == "male", ifelse(y > 18, "Adult male", "Underage male"),
ifelse(y > 18, "Adult female", "Underage female"))
"Underage male" "Underage male" "Adult female" "Adult male" "Adult female"
Now, suppose you want to set as 1 the individuals with more than 30 years or less than 10 and 0 in the other case. For that purpose, you can use the OR operator (|
).
ifelse (y > 30 | y <= 10, 1, 0)
1 0 1 1 0