The switch function in R
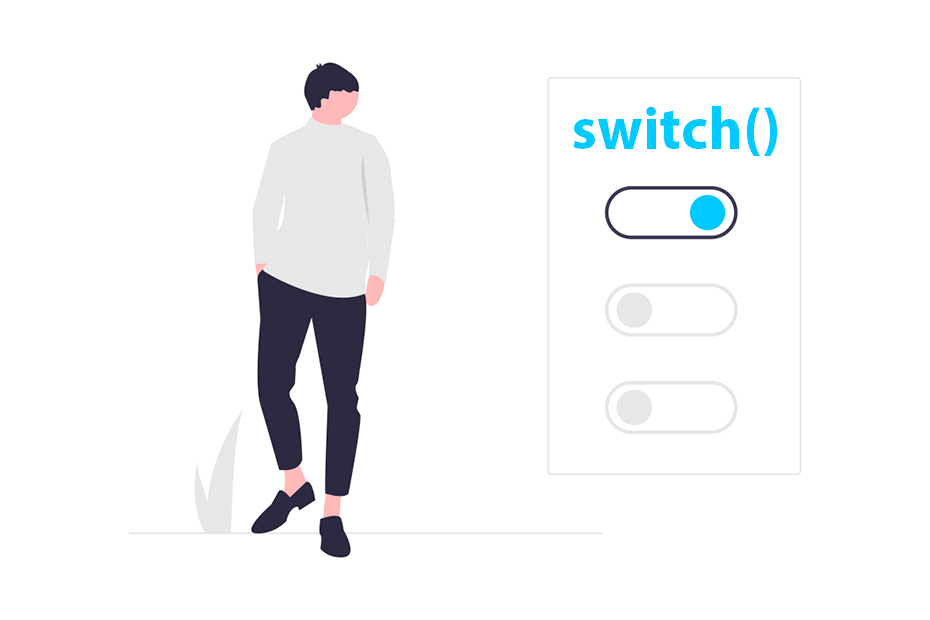
The switch
function evaluates a index or a string and chooses one of a list of alternatives. In this tutorial we will review the syntax and how to use this function with different examples.
Syntax and snippet of the switch
statement
The switch
function takes a number or a character string and a list of alternatives as input. The syntax of this function the following:
switch(EXPR, # Expression (Number or character)
... # List of alternatives (any object)
)
# Example:
switch(expression,
value1 = {
# Code if 'expression' is "value1"
},
value2 = {
# Code if 'expression' is "value2"
}
)
R also provides the following snippet to code faster with cases and actions:
switch(object, # Number or character
case = action # Cases and actions to take (names and objects)
)
The switch
function works in two different ways depending on whether the first argument is a number or a character string. We will review them in the examples below.
Examples
By index
If you input an integer and a list of objects, the switch
function will return the object placed on the corresponding index defined by the integer.
The following example returns "Second"
because it is the second element of the list of alternatives and the value passed as first argument was 2
.
value <- 2
switch(value, "First", "Second", "Third")
"Second"
Notice that you can input any object to the list of alternatives, such as vectors, matrices, data frames, etc. In the example below we return the third element of the list, which is a data frame.
value <- 3
switch(value, "A", 2:3, data.frame(x = 1:3, y = 2:4))
x y
1 1 2
2 2 3
3 3 4
If the integer is greater than the number of elements of the list of alternatives, then the function will return NULL
.
By name
If the value of EXPR
is a character string the string will be matched exactly to one of the names of the list of options and the corresponding object will be returned. In the following example we input "type_1"
, so that the object that corresponds to the first alternative will be returned.
value <- "type_1"
switch(value,
type_1 = "First",
type_2 = 2,
type_3 = "Third")
"First"
Now, if you input "type_2"
the function will match the second element and will return its corresponding object.
value <- "type_2"
switch(value,
type_1 = "First",
type_2 = 2,
type_3 = "Third")
2
Complex expressions
Notice that you can also add complex expressions inside each of the options adding your code inside curly braces. The following example calculates the square root of a number based on the selected option.
value <- "G_2"
switch(value,
"G_1" = {x <- 10
sqrt(x)
},
"G_2" = {x <- 9
sqrt(x)
},
)
3
Using switch
inside a function
The switch
statement can also be used inside a function. A common use case is to switch between different alternatives based on the value an argument takes.
In the following example we create a function that takes two numeric arguments (x
and y
) and other argument named method
that can take two values: sum
or mean
to calculate the sum or the mean of x
and y
, respectively. In addition, if you input a different string to method
, the function will throw an error.
# Sample function
fun <- function(x, y, method) {
switch(method,
"sum" = x + y,
"mean" = mean(c(x, y)),
stop('Input "sum" or "mean" to method')
)
}
# Examples
fun(5, 10, method = "sum") # 15
fun(5, 10, method = "mean") # 7.5
fun(5, 10, method = "mode") # Error in fun(5, 10, "mode") : Input "sum" or "mean" to method
R version 4.3.2 (2023-10-31 ucrt)