Data types in R
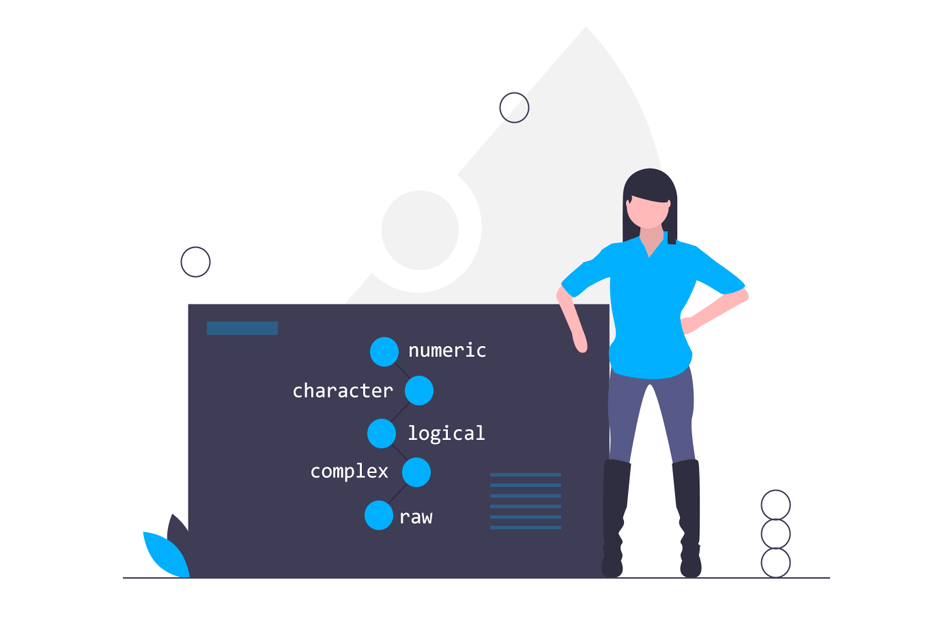
Everything in R programming language is an object. Hence, when we talk about data types in R we refer to the simplest data objects we can handle, that are also known as R atomic data types.
What are atomic data types in R?
Atomic data types are the object types which you can create (atomic) vectors with them. To clarify, the most common data types in R are the ones listed in the following list:
- Numeric: integer and double (real).
- Character.
- Logical.
- Complex.
- Raw.
Thus, you can check if any data object is atomic with the is.atomic
function. Note that this function checks for the data type of atomic vectors.
is.atomic(3) # TRUE
is.atomic("R CODER") # TRUE
In the following sections we will show how to check the data type of an object in R and explanations about each data type.
Check data type in R
There are several functions that can show you the data type of an R object, such as typeof
, mode
, storage.mode
, class
and str
.
# R internal type or storage mode of any object
typeof(1) # "double"
# Object class
class(2) # "numeric"
# Set or get the storage mode or type of an R object
# This classification is related to the S language
storage.mode(3) # "double"
mode(4) # "numeric"
# Object structure
str(5) # num 5
However, the main use of some of them is not to just check the data type of an R object. For instance, the class of an R object can be different from the data type (which is very useful when creating S3 classes) and the str
function is designed to show the full structure of an object. If you want to print the R data type, we recommend using the typeof
function.
x <- 1
class(x) # "numeric"
class(x) <- "My_class"
class(x) # "My_class"
typeof(x) # "double"
To summarize, the following table shows the differences of the possible outputs when applying typeof
, storage.mode
and mode
functions.
typeof | storage.mode | mode |
---|---|---|
logical | logical | logical |
integer | numeric | integer |
double | numeric | double |
complex | complex | complex |
character | character | character |
raw | raw | raw |
There are other functions that allow you to check if some object belongs to some data type, returning TRUE
or FALSE
. As a rule, these functions start with is.
followed by the data type. We will review all of them in the following sections.
Numeric data types
The numeric data type in R is composed by double (real) and integer data types. You can check if an object is numeric with the mode
function or if the is.numeric
function returns TRUE
.
mode(55) # "numeric"
is.numeric(3) # TRUE
Double or real data type
The double data type in R is the representation of a double-precision numeric object. Note that, by default, all numbers are double in R and that Inf
, -Inf
, NaN
, the scientific and hexadecimal notation of numbers are also doubles.
typeof(2) # "double"
# Infinite
typeof(Inf) # "double"
typeof(-Inf) # "double"
# Not a number
typeof(NaN) # "double"
# Scientific
typeof(3.12e3) # "double"
# Hexadecimal
typeof(0xbade) # "double"
If you want to check if an object is a double, you can use the is.double
function.
is.double(2) # TRUE
is.double(2.8) # TRUE
Integer data type
The integer data type can be created adding an L
to a number. This data type is useful if you want to pass some R object to a C or FORTRAN function that expects an integer value, so this data type it is not generally needed. You can check the data type with the is.integer
function.
y <- 2L
typeof(y) # "integer"
is.integer(3) # FALSE
is.integer(3L) # TRUE
Logical data type
The boolean or logical data type is composed by TRUE
, FALSE
and NA
values (missing values).
t <- TRUE
f <- FALSE
n <- NA
typeof(t) # "logical"
typeof(f) # "logical"
typeof(n) # "logical"
In addition, the function that prints whether an object is logical or not is is.logical
.
is.logical(T) # TRUE
is.logical(TRUE) # TRUE
Note that you can use T
and F
instead of TRUE
or FALSE
. However, the first is not recommended because you could override the value of T
or F
but not TRUE
or FALSE
, as shown in the following block of code.
F # FALSE
a <- T
# You can name a variable with T or F
F <- a
F # TRUE
# You can't name a variable with TRUE or FALSE
a <- TRUE
FALSE <- a # Error
Complex data type
The complex data type is an object that includes an imaginary number (i). As imaginary numbers are not generally used in statistics, this data type is not very common. The function to check the data type is is.complex
.
1 + 3i
typeof(1 + 3i) # "complex"
is.complex(1 + 3i) # TRUE
String or character data type
Character strings are symbols, letters, words or phases inside double or single quotation marks. With this in mind, you can verify that some object is of type character with the is.character
function.
character <- "a"
typeof(character) # "character"
is.character(character) # TRUE
Note that using single or double quotations marks is equivalent.
typeof('R CODER') # "character"
typeof("R CODER") # "character"
It is worth to mention that the nchar
function counts the number of characters inside a string, even the empty spaces.
nchar("A string") # 8
Raw data type in R
The raw data type holds raw bytes, so it is a very unusual data type. For instance, you could transform a character object or a integer numeric value to a raw object with the charToRaw
and intToBits
functions, respectively.
a <- charToRaw("R CODER")
a # 52 20 43 4f 44 45 52
typeof(a) # "raw"
b <- intToBits(3L)
typeof(b) # "raw"
As with the other data types, the function to verify the data type in this case is the is.raw
function.
is.raw(b) # TRUE
Data types coercion in R
You can coerce data types in R with the functions starting with as.
, summarized in the following table:
Function | Coerced data type |
---|---|
as.numeric | Numeric |
as.integer | Integer |
as.double | Double |
as.character | Character |
as.logical | Boolean |
as.raw | Raw |
Consider you want to coerce a double to integer, you could do as follows:
a <- 3
typeof(a) # "double"
a <- as.integer(a)
typeof(a) # "integer"
Moreover, you could also coerce a logical value to numeric (0 and 1) or a character string:
b <- TRUE
b <- as.numeric(b)
b # 1
c <- FALSE
c <- as.numeric(c)
c # 0
d <- TRUE
d <- as.character(d)
d # "TRUE"
Nevertheless, if you try to coerce two non-compatible data types (like a character string to numeric) an error will arise:
as.double("R CODER")
NA
Warning message: NAs introduced by coercion