Export data from R
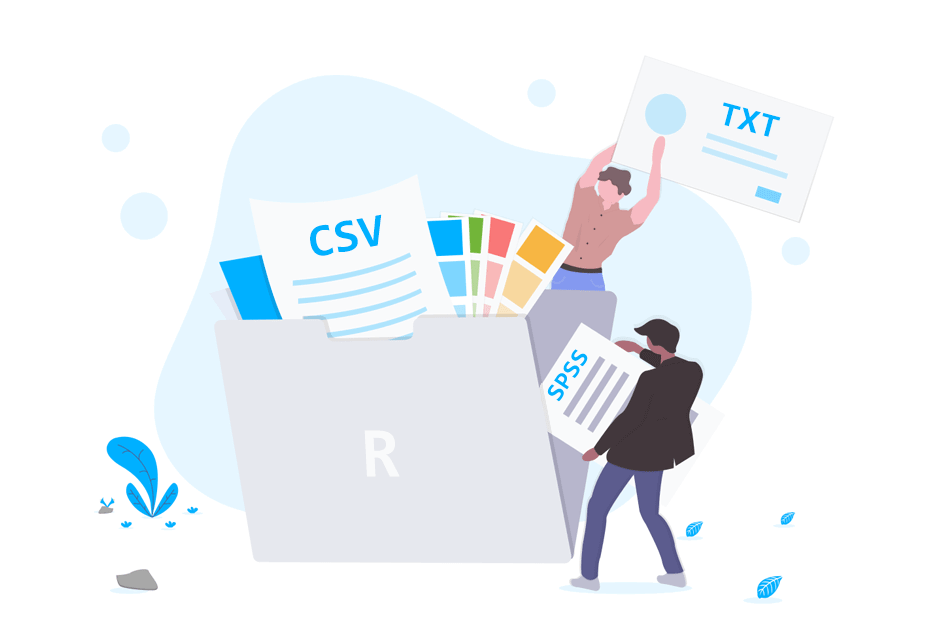
R allows you to work with data and store it in variables in the workspace. However, sometimes you need you export or save to share or work with the results in other software. In this tutorial you will learn how to export data in R or RStudio. Note that you can export data from R to several formats, like CSV, SAV, XLS, XLSX, TXT or even XML.
Save R objects
When working with R, you can save the objects stored in your workspace. There are three main options, depending on if you want to save the full workspace, some objects or just one.
Exporting R objects from your workspace is very useful when your code takes a considerable amount of time to run, like in simulation studies. In consequence, if you need the results after the first execution you won’t need to run the code again.
First, you can export the full workspace from R with the save.image
function as shown in the following block of code. Note that the output file will be of type RData
.
# Export all objects (the workspace image)
save.image(file = "R_objects.RData")
Second, if you just need to export some objects you can specify them separated by comma with the save
function.
# Export some R objects
save(x, y, file = "Two_Objects.RData")
Finally, to save only one object it is more recommended saving it as RDS
with the saveRDS
function:
# Export just one R object
saveRDS(x, file = "One_Object.rds")
If you specify compress = TRUE
as argument of the previous functions the file will be compressed by default as gzip.
Save data frame as CSV in R
In case you want to export a data frame as CSV in R, you can make use of the write.csv
or write.csv2
functions.
The use of one or the other will depend on the format of your data. In some countries they use a comma as decimal separator, so you can’t save a CSV separated by commas in this scenario. In this case, the CSV uses a semi-colon as separator of the data. The last is what the write.csv2
function does, while the write.csv
uses a comma to separate the data. In consequence, you can export to CSV typing:
df <- data.frame(x = rnorm(10), y = rnorm(10))
# Comma as separator and dot as decimal separator
write.csv(df, "my_df.csv")
# Semi-colon as separator and comma as decimal separator
write.csv2(df, "my_df.csv")
Note you can also write a table to CSV in R with the same functions:
tb <- table(chickwts$feed)
write.csv(tb, "my_tb.csv")
write.csv2(tb, "my_tb.csv")
Recall to type ?write.csv
or help(write.csv)
for further information.
In this and the other functions of this tutorial, if you specify only the file name, the file will be saved in the working directory. If you want other location, change the working directory or specify the full path, instead of only the name of the file.
Export data from R to TXT file
In order to export a TXT in R you can use the write.table
function. Consider, for instance, that you want to export a data frame from R to a TXT file. In this case you could write:
df <- trees[1:5, ]
write.table(df, "file.txt")
However, if you want to print to the console what you are going to save, before saving it, you can type:
write.table(df)
"Girth" "Height" "Volume"
"1" 8.3 70 10.3
"2" 8.6 65 10.3
"3" 8.8 63 10.2
"4" 10.5 72 16.4
"5" 10.7 81 18.8
Note that you can also export an R object, like a vector, to TXT with this function:
x <- runif(5)
write.table(x, "vector.txt")
If you save as TXT an R object different than a data frame or matrix, it will be coerced to a data frame.
In addition, there are several arguments you can customize. As an example, if you want to specify the encoding and to write the table without quotes you can type:
write.table(x, "vector.txt", fileEncoding = "UTF-8", quote = FALSE)
Export data from R to Excel (XLS and XLSX)
Exporting data from R to Excel can be achieved with several packages. The most known package to export data frames or tables as Excel is xlsx
, that provides the write.xlsx
and write.xlsx2
functions.
# Requires JAVA
# install.packages("xlsx")
library(xlsx)
# Comma as separator and dot as decimal separator
write.xlsx(x, # Data frame to be exported
file, # Full path
sheetName = "Sheet1", # Name of the sheet
col.names = TRUE, # Whether to include column names or not
row.names = TRUE, # Whether to include row names or not
append = FALSE, # Whether to append to an existing file
showNA = TRUE, # If TRUE, NA are empty cells
password = NULL) # Password as string
# Semi-colon as separator and comma as decimal separator
write.xlsx2(x, file, sheetName = "Sheet1", col.names = TRUE,
row.names = TRUE, append = FALSE, showNA = TRUE, password = NULL)
As an example, if you want to save the cars
dataset you can type:
write.xlsx(cars, "cars.xlsx")
However, the main disadvantage of the previous package is that requires JAVA installed on your computer, so if the JAVA_HOME
is not specified or if R doesn’t find the rJava.dll
file, the package won’t work.
Alternatives to export R output to XLSX that don’t require JAVA are the openxlsx
and writexl
packages:
On the one hand, the write.xlsx
function of the openxlsx
allows you to write a data frame or list of data frames to an XLSX file:
# install.packages("openxlsx")
library(openxlsx)
write.xlsx(data_frame, "file_name.xlsx")
On the other hand, the writexl
library provides the write_xlsx
function to export data frames in R to Excel:
# install.packages("writexl")
library("writexl")
write_xlsx(data_frame, "file_name.xlsx")
Export to SPSS from R
In case you want to export to SPSS from R you will need to install the package named haven
, that contains the write_sav
function. The process of saving the data is analogous to the others:
# install.packages("haven")
library(haven)
write_sav(mtcars, "mtcars.sav")
Save to STATA from R
In case you want export data from R to STATA, you will need to use the write.dta
function of the foreign
package as follows:
# install.packages("foreign")
library(foreign)
write.dta(data_frame, "my_data.dta")
Export data from R to XML
To easily export a dataframe to XML format you can use the write.xml
function of the kulife
package, indicating as first argument the dataset and the name of the exported file in the second. In this example we are going to save the mtcars
dataset.
# install.packages("kulife")
library(kulife)
write.xml(mtcars, "mtcars.xml")
<?xml version="1.0"?>
<document>
<row>
<mpg>21</mpg>
<cyl>6</cyl>
<disp>160</disp>
<hp>110</hp>
<drat>3.9</drat>
<wt>2.62</wt>
<qsec>16.46</qsec>
<vs>0</vs>
<am>1</am>
<gear>4</gear>
<carb>4</carb>
</row>
Nonetheless, you might have noticed that the XML output file does not contain the row names of the data frame, as the markup language doesn’t support naming the rows. To solve this issue, in this example you can bind the row names to the dataset and name the new column as “car”.
data <- cbind(rownames(mtcars), mtcars)
colnames(data)[1] <- "car"
write.xml(data, "mtcars.xml")
<?xml version="1.0"?>
<document>
<row>
<car>Mazda RX4</car>
<mpg>21</mpg>
<cyl>6</cyl>
<disp>160</disp>
<hp>110</hp>
<drat>3.9</drat>
<wt>2.62</wt>
<qsec>16.46</qsec>
<vs>0</vs>
<am>1</am>
<gear>4</gear>
<carb>4</carb>
</row>
Now, each row of the XML document will contain the name of the car and its characteristics.