Row and column names in R
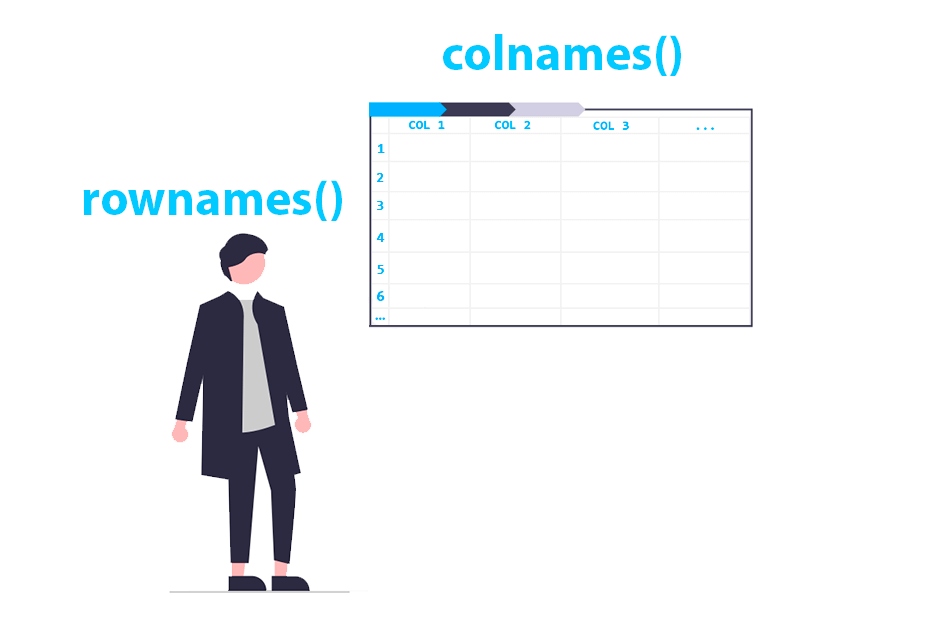
The rownames
and colnames
functions allow you to get and set row and column names for matrix-like objects, such as data frames or matrices. The dimnames
is similar, as it allows you to retrieve or set both the column and row names of an object.
Get and set row names with rownames
The rownames
function allows you to retrieve the row names of a data frame or matrix. The syntax of the function is the following:
# Get row names
rownames(x)
# Set row names to "value" (usually a vector or NULL)
rownames(x) <- value
In the following block of code we create a sample data frame with two columns and ten rows. The data frame looks like this:
df <- data.frame(col1 = 1:10, col2 = 11:20)
df
col1 col2
1 1 11
2 2 12
3 3 13
4 4 14
5 5 15
6 6 16
7 7 17
8 8 18
9 9 19
10 10 20
You can check the default row names with the rownames
function. As the row names hasn’t been set yet, the default names are the index of each row.
rownames(df)
"1" "2" "3" "4" "5" "6" "7" "8" "9" "10"
Nonetheless, the rownames
function can also be used to set the row names. For that purpose, you will need to assign the row names a vector with as many elements as rows.
rownames(df) <- paste("Row", 1:10)
rownames(df)
"Row 1" "Row 2" "Row 3" "Row 4" "Row 5" "Row 6" "Row 7" "Row 8" "Row 9" "Row 10"
Now, if you check the sample data set you will see that the rows have the name you set with the previous code.
df
col1 col2
Row 1 1 11
Row 2 2 12
Row 3 3 13
Row 4 4 14
Row 5 5 15
Row 6 6 16
Row 7 7 17
Row 8 8 18
Row 9 9 19
Row 10 10 20
Get and set column names with colnames
The colnames
function behaves the same as rownames
but for column names. The syntax is as follows:
# Get column names
colnames(x)
# Set column names to "value" (usually a vector or NULL)
colnames(x) <- value
If you want to check the column names of your object you just need to input the name of your object to the function.
colnames(df)
"col1" "col2"
You can also customize the column names of your object by assigning a new values to the column names.
colnames(df) <- c("Column 1", "Column 2")
colnames(df)
"Column 1" "Column 2"
Now you can check that your data frame has the new column names:
df
Column 1 Column 2
Row 1 1 11
Row 2 2 12
Row 3 3 13
Row 4 4 14
Row 5 5 15
Row 6 6 16
Row 7 7 17
Row 8 8 18
Row 9 9 19
Row 10 10 20
The dimnames
function
The dimnames
function can be used to retrieve both row and column names of an object. The output is a list where the first element are the row names and the second the column names.
dimnames(df)
[[1]]
[1] "Row 1" "Row 2" "Row 3" "Row 4" "Row 5" "Row 6" "Row 7" "Row 8" "Row 9" "Row 10"
[[2]]
[1] "Column 1" "Column 2"
You can access each of them by accessing each element of the list as in the example below.
# Row names
dimnames(df)[[1]]
# Column names
dimnames(df)[[2]]
"Row 1" "Row 2" "Row 3" "Row 4" "Row 5" "Row 6" "Row 7" "Row 8" "Row 9" "Row 10"
"Column 1" "Column 2"
If you want to change the row and column names you can assign a new value with dimnames(x)[[1]] <- value
or dimnames(x)[[2]] <- value
, but it is recommended to use the rownames
and colnames
functions instead.
Remove column and row names
Sometimes you just want to remove the column and/or row names of your matrix or data frame. For that purpose you will need to assign the columns and/or rows to NULL
.
# Remove row names
rownames(df) <- NULL
# Remove column names
colnames(df) <- NULL
# Check data frame
df
Now the data frame doesn’t have row or column names.
1 1 11
2 2 12
3 3 13
4 4 14
5 5 15
6 6 16
7 7 17
8 8 18
9 9 19
10 10 20