rbind() and cbind() functions in R
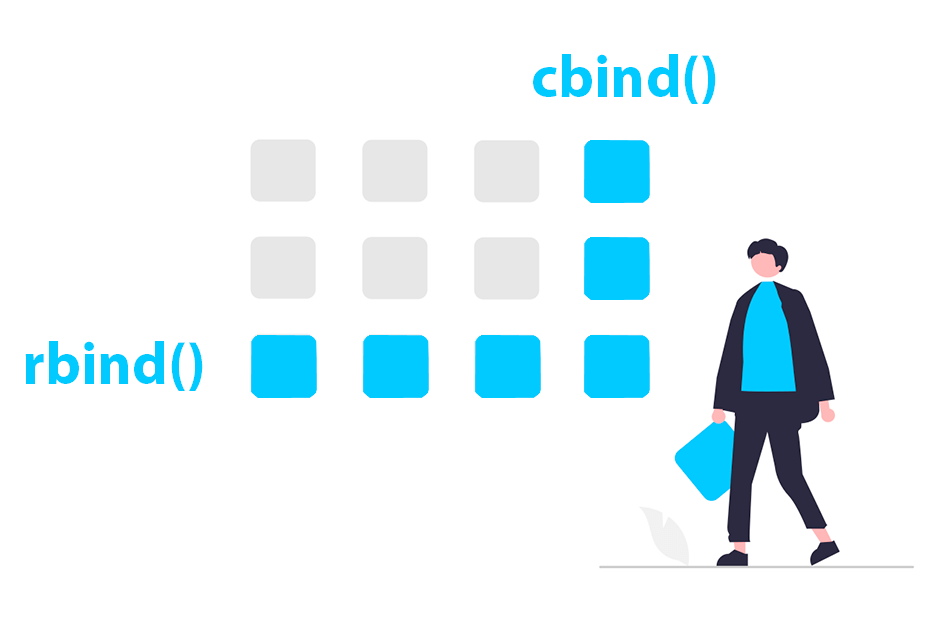
The rbind
function combines vectors, matrices or data frames by rows while the cbind
function combines them by columns. In this tutorial you will learn how to use these functions in several use cases.
Binding rows with rbind
If you want to combine two or more vectors of the same length by binding them by rows you can input them to the rbind
function. The output will be a matrix with as many rows as vectors and as many columns as elements.
x <- c(1, 2, 3)
y <- c("G1", "G2", "G3")
# Bind two vectors by rows
rbind(x, y)
[,1] [,2] [,3]
x "1" "2" "3"
y "G1" "G2" "G3"
If the length of the vectors is not equal you will get a warning message indicating that the ânumber of columns of result is not a multiple of vector length (arg 1)â. In this scenario the elements of the smaller vectors will be recycled.
However, you can bind a single value (numeric or character) with a vector and the value will be recycled to the length of the largest vector.
x <- c(1, 2, 3)
# Bind integer and vector
rbind(2, x)
[,1] [,2] [,3]
2 2 2
x 1 2 3
Add new rows to a data frame or matrix
Consider the following sample data frame with three rows and two columns:
df <- data.frame(x = 1:3, y = c("G1", "G2", "G3"))
df
x y
1 1 G1
2 2 G2
3 3 G3
If you want to bind a new row to it you can input the data frame and the vector to rbind
, as shown below.
df <- data.frame(x = 1:3, y = c("G1", "G2", "G3"))
z <- c(4, "G4")
# Add a new row
df <- rbind(df, z)
df
x y
1 1 G1
2 2 G2
3 3 G3
4 4 G4
Note that you can also input several vectors to the function to bind several rows to the data frame.
df <- data.frame(x = 1:3, y = c("G1", "G2", "G3"))
z <- c(4, "G4")
w <- c(5, "G5")
# Add TWO new rows
df <- rbind(df, z, w)
df
x y
1 1 G1
2 2 G2
3 3 G3
4 4 G4
5 5 G5
Bind data frames by row
You can also bind two or more data frames together with the rbind
function as long as they have the same number of columns and column names.
df1 <- data.frame(x = 1:3, y = c("G1", "G2", "G3"))
df2 <- data.frame(x = 15:19, y = c("G15", "G16", "G17", "G18", "g19"))
# Bind two data frames
df <- rbind(df1, df2)
df
x y
1 1 G1
2 2 G2
3 3 G3
4 15 G15
5 16 G16
6 17 G17
7 18 G18
8 19 g19
Bind data frames with different column names
Notice that if the names of the columns of the data frames are not the same you wonât be able to merge them despite there is a common column name. An error will arise in this scenario.
df1 <- data.frame(x = 1:3, y = c("G1", "G2", "G3"))
df2 <- data.frame(x = 15:19, z = c("Z1", "Z2", "Z3", "Z4", "Z5"))
# Bind two data frames
df <- rbind(df1, df2)
df
Error in match.names(clabs, names(xi)) :
names do not match previous names
In case that you need to bind two data frames by rows with different column names or with only some common column names you will need to use the bind_rows
function from dplyr
.
df1 <- data.frame(x = 1:3, y = c("G1", "G2", "G3"))
df2 <- data.frame(x = 15:18, z = c("Z1", "Z2", "Z3", "Z4"))
# Bind two data frames with different column names
library(dplyr)
df <- dplyr::bind_rows(df1, df2)
df
x y z
1 1 G1 <NA>
2 2 G2 <NA>
3 3 G3 <NA>
4 15 <NA> Z1
5 16 <NA> Z2
6 17 <NA> Z3
7 18 <NA> Z4
Row labels when binding rows
The rbind
function provides an argument named deparse.level
which defaults to 1
. If you set this argument to 0
the output will have no row labels and when set to 2
the labels will be constructed from the argument names.
x <- c(1, 2, 3)
# Bind integer and vector
rbind(2, x, deparse.level = 0)
[,1] [,2] [,3]
[1,] 2 2 2
[2,] 1 2 3
Binding columns with cbind
The cbind
function binds vectors, data frames or matrices by columns. You can combine several vectors of the same length by columns passing them as input to cbind
. The function will return a matrix with as many columns as vectors and as many rows as elements of the vectors.
x <- c(1, 2, 3)
y <- c("G1", "G2", "G3")
# Bind two vectors by columns
cbind(x, y)
x y
[1,] "1" "G1"
[2,] "2" "G2"
[3,] "3" "G3"
You can also bind a single numeric or character value with other object. In this scenario the value will be repeated to fit the length of the other object.
x <- c(1, 2, 3)
# Bind a character and a vector
cbind("A", x)
x
[1,] "A" "1"
[2,] "A" "2"
[3,] "A" "3"
Add new columns
The function allows you to add columns to a data frame or matrix passing as input the data frames and the new columns.
df <- data.frame(a = c("A", "B", "C"), b = c(1, 2, 3))
x <- c("AA", "BB", "CC")
# Add a new column
cbind(df, x)
x y
[1,] "1" "G1"
[2,] "2" "G2"
[3,] "3" "G3"
Combining data frames
In addition, you can combine several data frames as long as they have the same number of rows, otherwise the function will throw an error.
df1 <- data.frame(a = c("A", "B", "C"), b = c(1, 2, 3))
df2 <- data.frame(c = c("D", "E", "F"), d = c(4, 5, 6))
# Combine two data frames
cbind(df1, df2)
a b c d
1 A 1 D 4
2 B 2 E 5
3 C 3 F 6
If the number of rows of the data frames is not the same you will get an error indicating that âarguments imply differing number of rowsâ.
Column labels when binding columns
The deparse.level
argument from cbind
determines the column labels. By default (1
) the function will use the column names of the objects, if any, if 0
the output wonât have column names and if 2
the function will always construct column names from the input values.
x <- c(1, 2, 3)
# Bind an integer and a vector
cbind(3, x, deparse.level = 0)
[,1] [,2]
[1,] 3 1
[2,] 3 2
[3,] 3 3