Rename columns in R with the rename() function from dplyr
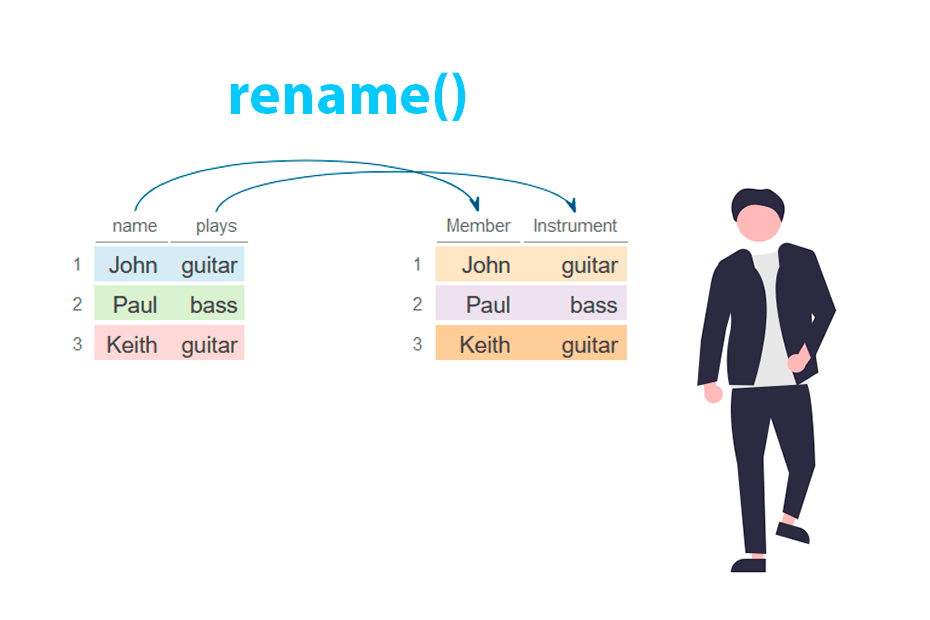
The rename
function from dplyr can be used to alter column names of a data frame. In addition, rename_with
allows to rename columns using a function.
Syntax
The rename
function alters column names using the syntax new_name = old_name
while rename_with
takes a function (.fn
) to transform the column names from a set of columns (.cols
, all by default).
# Set new column names
rename(.data, new_name = old_name)
# Rename the selected columns (all by default) based on a function
rename_with(.data, .fn, .cols)
Rename a single column
For this tutorial we will utilize the band_instruments
dataset from dplyr
, which includes two columns named name
and plays
.
Considering that you want to rename the first column to "First Name"
you can execute the following command:
library(dplyr)
# Rename 'name' as 'First Name'
df_2 <- band_instruments %>%
rename("First Name" = name)
df_2
`First Name` plays
<chr> <chr>
1 John guitar
2 Paul bass
3 Keith guitar
Notice that you can also rename columns by index. The following example illustrates how to rename the second column of the data set.
library(dplyr)
# Rename the second column as 'Second column'
df_2 <- band_instruments %>%
rename("Second column" = 2)
df_2
# A tibble: 3 × 2
name `Second column`
<chr> <chr>
1 John guitar
2 Paul bass
3 Keith guitar
Rename multiple columns
It is possible to rename multiple columns at once by adding more new_name = old_name
expressions to the function separated by comma. The following renames the column name
as Member
and the column plays
as Instrument
.
library(dplyr)
# Rename 'name' as 'Member'
# Rename 'plays' as 'Instrument'
df_2 <- band_instruments %>%
rename("Member" = "name",
"Instrument" = "plays")
df_2
# A tibble: 3 × 2
Member Instrument
<chr> <chr>
1 John guitar
2 Paul bass
3 Keith guitar
An alternative to the previous is to use a named vector with the old names and new names in conjunction with all_of
or any_of
. The difference is that any_of
won’t throw an error if any “old name” is not on the dataset.
library(dplyr)
# Rename 'name' as 'Member name' and 'plays' as 'Instrument'
names <- c("Member name" = "name", "Instrument" = "plays")
df_2 <- band_instruments %>%
rename(all_of(names)) # Or any_of(names)
df_2
# A tibble: 3 × 2
`Member name` Instrument
<chr> <chr>
1 John guitar
2 Paul bass
3 Keith guitar
Rename columns using a function with rename_with
The rename_with
function renames all or a set of columns based on a function.
Rename all columns
By default, the rename_with
function will rename all columns based on the input function. In the example below, we use the toupper
function to rename all the columns in uppercase.
library(dplyr)
# Rename all the columns with the names in uppercase
df_2 <- band_instruments %>%
rename_with(toupper)
df_2
# A tibble: 3 × 2
NAME PLAYS
<chr> <chr>
1 John guitar
2 Paul bass
3 Keith guitar
If the input function takes more arguments, such as paste or paste0 functions you will need to add ~
before the function and a dot .
to represent the column names. In the following example we also set recycle0 = TRUE
to recycle empty selections if needed.
library(dplyr)
# Add a prefix to the existing columns
df_2 <- band_instruments %>%
rename_with(~paste0("A_", ., recycle0 = TRUE))
df_2
# A tibble: 3 × 2
A_name A_plays
<chr> <chr>
1 John guitar
2 Paul bass
3 Keith guitar
Note that you can also input custom function as the following, which adds "New_"
as prefix of each column name.
library(dplyr)
custom_function <- function(x) {
paste0("New_", x)
}
# Add a prefix to the existing columns
df_2 <- band_instruments %>%
rename_with(custom_function)
df_2
# A tibble: 3 × 2
New_name New_plays
<chr> <chr>
1 John guitar
2 Paul bass
3 Keith guitar
Rename specific columns
By default .cols = everything()
, which means that the input function is applied to all the columns of the data. However, you can select the desired columns by using helper functions such as contains
, starts_with
, ends_with
etc. See the full list of helper functions to select columns.
library(dplyr)
# Column names containing the string "nam" to upper case
df_2 <- band_instruments %>%
rename_with(toupper, .cols = contains("nam"))
df_2
# A tibble: 3 × 2
NAME plays
<chr> <chr>
1 John guitar
2 Paul bass
3 Keith guitar