Concatenate strings in R with paste and paste0
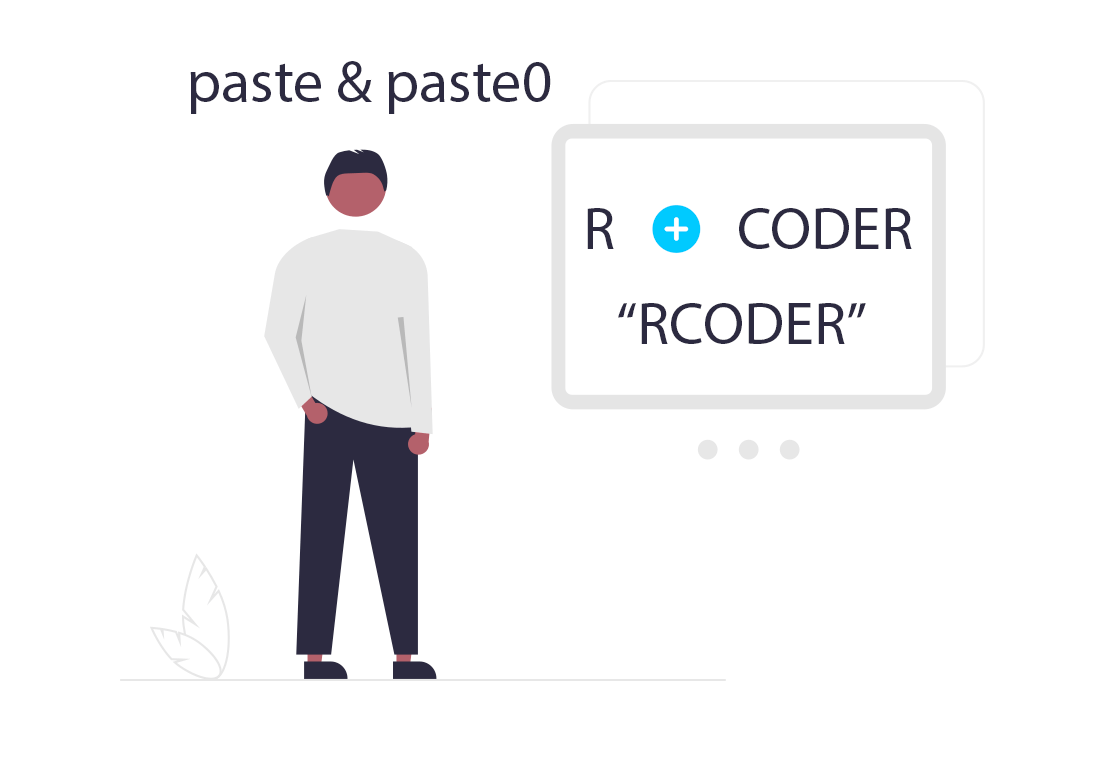
The paste
and paste0
functions are the R functions used to concatenate strings. The main difference between both functions is that paste
concatenates strings separated by an empty space (by default), while paste0
concatenates strings without any space.
The paste0
function
The paste0
function allows to concatenate two or more strings into one without spaces, so if you need to add spaces use the paste function or add a space to the strings when needed. Keep in mind that you can also input numbers that will be converted to character through the as.character function.
In the example below we are concatenating two strings together in order to create a new string. Note that we added a space at the beginning of the second string to separate the words.
paste0("First string", " second string")
"First string second string"
The function is also useful to concatenate results passing a variable to the function. In the following example we are concatenating a string and a value stored on a variable.
x <- 12
paste0("The value is: ", x)
"The value is: 12"
Pasting vectors
The function also works with vectors. Note that the elements of the vectors will be concatenated term by term. In the following block of code we are concatenating a vector from 1 to 10 with an exclamation sign.
paste0(1:10, "!")
"1!" "2!" "3!" "4!" "5!" "6!" "7!" "8!" "9!" "10!"
You can also paste two vectors together even if they are not of the same length. The final length will be the length of the longest vector and the shortest vector will repeat its pattern.
paste0(1:10, LETTERS[1:15])
"1A" "2B" "3C" "4D" "5E" "6F" "7G" "8H" "9I" "10J" "1K" "2L" "3M" "4N" "5O"
Collapse
The paste0
function provides an argument named collapse
that allows to separate the results when concatenating a vector. For instance, considering that you want to separate your results by a comma you will need to set collapse = ", "
.
paste0(1:10, "!", collapse = ", ")
"1!, 2!, 3!, 4!, 5!, 6!, 7!, 8!, 9!, 10!"
The paste
function
The paste
command behaves the same way as paste0
, but the former will add a space by default when concatenating strings that can be override with other string making use of the sep
argument. Consider that you want to concatenate the letter A with the letter B, the result will be as follows:
paste("A", "B")
"A B"
Custom separator
However, you can also customize the separator with any other string. In the following example we add a plus sign as separator. This can be useful to create formulas for linear regression models.
paste("A", "B", sep = "+")
"A+B"
Collapse to new line
The paste
function also provides the collapse
argument that behaves the same way as with paste0
. In the following example we are setting collapse = "\n"
so if the string is used on as the title or text of a plot, each element of the paste will be on a new line.
paste(c("x =", "y =", "z ="), 3:5, collapse = "\n")
"x = 3\ny = 4\nz = 5"
paste0(..., collapse)
is equivalent to paste(..., sep = "", collapse)
, but paste0
is slightly more efficient in this case.
If you want to remove the quotes from the output of paste0
you can use the noquote
function.