Number of rows and columns in R
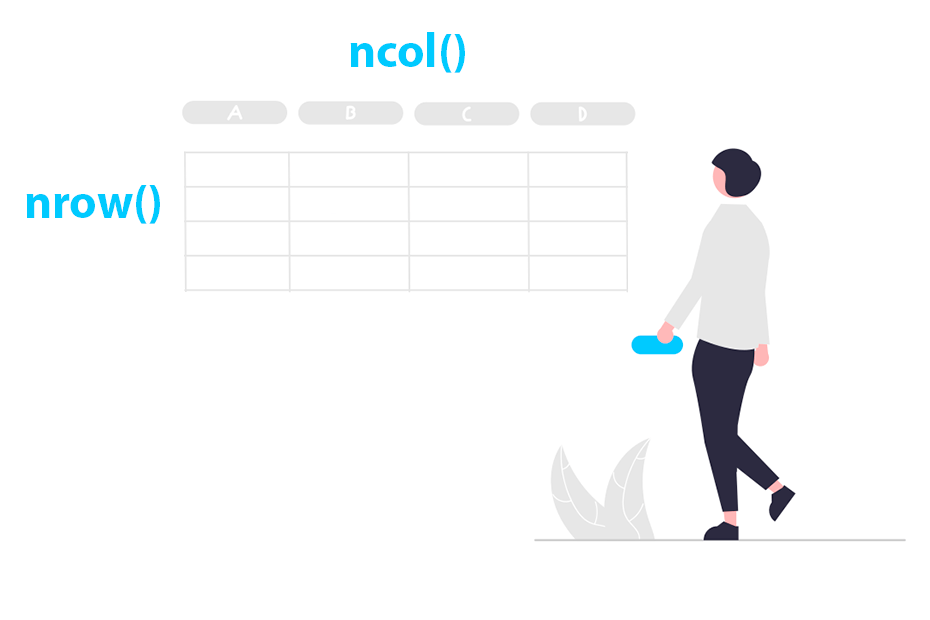
Return the number of rows or columns of a data frame or matrix with the nrow
, ncol
functions and learn the difference between them and the NCOL
and NROW
functions. You will also learn how to return or set both the rows and columns with dim
.
Number of columns with ncol
Consider the following data frame named df
:
df <- data.frame(x = 1:5, y = LETTERS[1:5])
# Print df
df
x y
1 1 A
2 2 B
3 3 C
4 4 D
5 5 E
Inspecting the data frame you can see that it has two columns, but if it has lots of columns or you want to get that number programmatically you can use the ncol
function passing the data frame as input:
ncol(df)
2
The ncol
function also provides the number of columns of a matrix. Consider the following sample matrix named m
.
m <- matrix(data = 1:15, ncol = 5)
# Print matrix
m
[,1] [,2] [,3] [,4] [,5]
[1,] 1 4 7 10 13
[2,] 2 5 8 11 14
[3,] 3 6 9 12 15
You can get the number of columns of m
passing the matrix as input to the function. In this case the matrix has 5 columns.
ncol(m)
5
R provides a function named NCOL
which do the same as ncol
but treats vectors as a one column matrix. This means that if you apply ncol
to a vector you will get a NULL
value but if you apply NCOL
you will get a 1.
x <- 1:5
ncol(x) # NULL
NCOL(x) # 1
ncol(NULL) # NULL
NCOL(NULL) # 1
Number of rows with nrow
Consider the same data frame of the previous section:
df <- data.frame(x = 1:5, y = LETTERS[1:5])
# Print df
df
x y
1 1 A
2 2 B
3 3 C
4 4 D
5 5 E
You can get the number of row passing the data frame as input. In this case the number of rows of the data frame is 5.
nrow(df)
5
The function also works for matrices. Consider the following sample matrix:
m <- matrix(data = 1:15, ncol = 5)
# Print matrix
m
[,1] [,2] [,3] [,4] [,5]
[1,] 1 4 7 10 13
[2,] 2 5 8 11 14
[3,] 3 6 9 12 15
If you want to get the number of rows of the previous matrix you can use the nrow
function as follows. In this example the matrix has 3 rows.
nrow(m)
3
R also provides a function named NROW
which do the same as nrow
but treats vectors as a one column matrix, so each element of the the vector will be treated as a row. This means that if you apply nrow
to a vector you will get a NULL
value but if you apply NROW
you will get the number of elements of the vector.
x <- 1:5
nrow(x) # NULL
NROW(x) # 5
nrow(NULL) # NULL
NROW(NULL) # 0
Get or set the dimensions
The dim
function can be used to return the dimensions of an object, this is, both the number of rows and the number of columns.
df <- data.frame(x = 1:5, y = LETTERS[1:5])
dim(df)
5 2
The first element of the output is the number of rows and the second the number of columns of the sample data frame.
However, this function can also be used to set the dimensions of an object. Consider the following sample vector:
x <- 1:10
# Print x
x
1 2 3 4 5 6 7 8 9 10
If you apply the dim
function you will get a NULL
, as the object doesn’t have dimensions.
dim(x)
NULL
Nonetheless, you can set the dimensions assigning a vector with the desired dimensions. In the example below we set 2 rows and 5 columns, so the vector is transformed into a matrix.
dim(x) <- c(2, 5)
# Print x
x
[,1] [,2] [,3] [,4] [,5]
[1,] 1 3 5 7 9
[2,] 2 4 6 8 10
Now you can check that the dimensions of the object are those specified:
dim(x)
2 5