Try catch in R
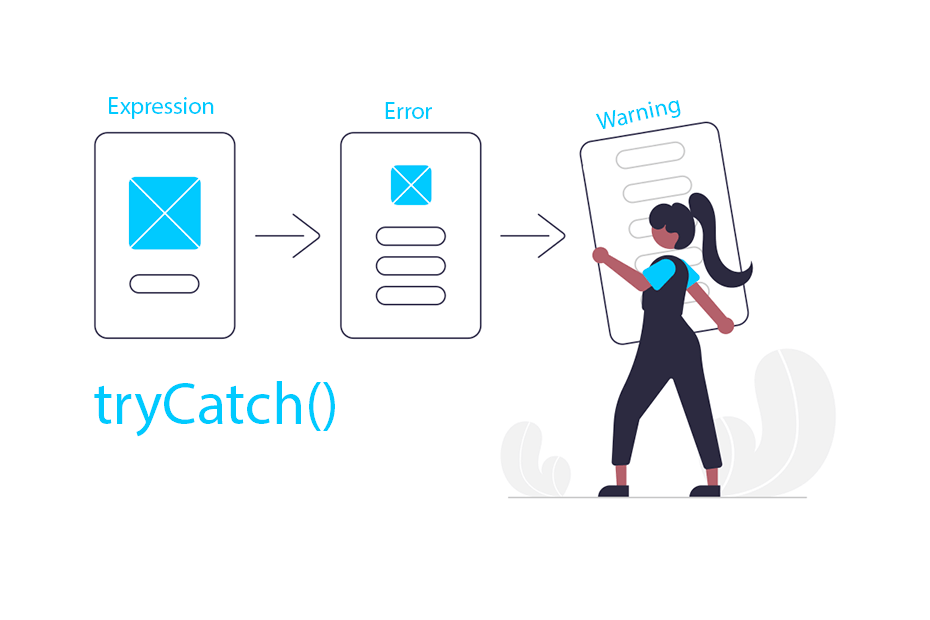
The tryCatch
function allows to catch errors and warnings when running an R code and handle them the way you want. This will allow you to evaluate some code when an error or a warning arises or just show the error or warning message, avoiding the code to stop or getting incorrect results.
Syntax
The syntax of a tryCatch
in R is the following:
tryCatch(
expr = {
# Expression to be evaluated
},
error = function(e){
# Do something to handle error
# print(e)
},
warning = function(w){
# Do something to handle warning
# print(w)
},
finally = {
# Expression to be evaluated before returning or exiting
}
)
The main code goes inside expr
, if an error is catch the code inside error
will be evaluated and if a warning is catch the code inside warning
will be evaluated. The code inside finally
will be evaluated regardless if there is any error or warning.
Note that error
, warning
and finally
are optional, so you can only specify one or two if desired.
Error handling
Consider that you are writing a function that might arise an error and you don’t want your code to stop. In this situation your best choice is to use tryCatch
and evaluate some code if the error arises.
In the following example we are multiplying the input value by two, but if an error is catch, the error will be printed, the input value will be coerced to numeric and then multiplied by two and returned.
# Sample function
test_fun <- function(x) {
tryCatch(
# Code to be evaluated
expr = {
x <- x * 2
return(x)
},
# Code if an error is catch
error = function(e){
print(e)
x <- as.numeric(x) * 2
return(x)
}
)
}
If you try the function with a numeric input, no error will arise and the function will return the value multiplied by two:
res <- test_fun(10)
res
20
However, if the input value is a string, an error will arise because the value can’t be multiplied, so the code inside error
will be evaluated. The error will be printed, the input will be coerced to numeric and the desired result will be calculated.
res <- test_fun("10")
res
<simpleError in x * 2: non-numeric argument to binary operator>
20
If you are evaluating some code inside a loop and an error arise the code won’t stop if you catch it with tryCatch
. Note that you can just print the error or some message to be aware of the iteration that failed and take no further action if you don’t want to.
Warning handling
If the evaluated expression can throw a warning message and you want to catch it and evaluate some code if that happens you can handle it with warning
.
The example below is similar to the previous, but in this scenario we try to catch a warning that happens when a character cannot be coerced to numeric, so the warning message and a custom message will be printed and the function will return an NA
.
# Sample function
test_fun_2 <- function(x) {
tryCatch(
# Code to be evaluated
expr = {
x <- as.numeric(x)
x <- x * 2
return(x)
},
# Code if a warning is catch
warning = function(w){
print(w)
message("Input a numeric value")
return(NA)
}
)
}
If you try to input a character that cannot be coerced to numeric (such as "A"
) the as.numeric
function will throw a warning message and the code inside warning
will be evaluated.
res <- test_fun_2("A")
res
<simpleWarning in doTryCatch(return(expr), name, parentenv, handler): NAs introduced by coercion>
Input a numeric value
NA
Evaluate expression before exiting
If you want to evaluate some expression despite the code throws an error or a warning you can use finally
. In the example below we are creating a function that multiplies a number by two and when the code is evaluated, a message with “Done” is shown on console.
# Sample function
test_fun_3 <- function(x) {
tryCatch(
# Code to be evaluated
expr = {
x <- x * 2
return(x)
},
# Code to be evaluated before returning or exiting
finally = {
message("Done")
}
)
}
If you test the function you will see the result and the message.
res <- test_fun_3(5)
res
Done
10