Square root in R
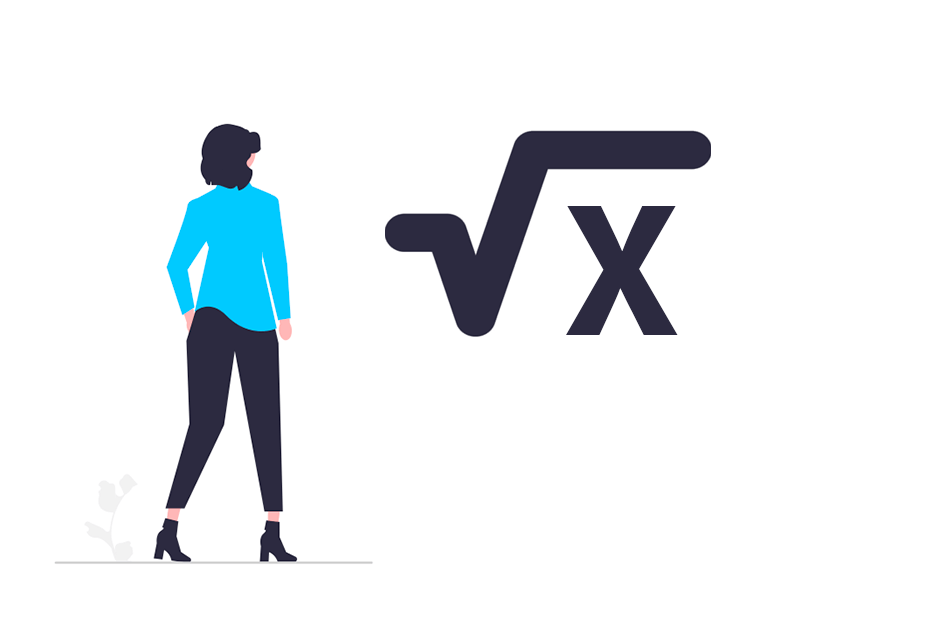
The square root of a number is other number that multiplied by itself gives the first number. In R, you can make use of the sqrt
function to compute the square root for a numeric value or vector.
Square root
If you want to calculate the square root of a number you just need to input that number to the sqrt
function as follows:
sqrt(8)
2.828427
Keep in mind that you can also input a numeric vector, so the function will return the square root for each of the elements of that vector.
sqrt(c(10, 8, 16, 25))
3.162278 2.828427 4.000000 5.000000
The input value must be numeric or an error will arise indicating âError in sqrt(âxâ) : non-numeric argument to mathematical functionâ. If this error happens you will need to transform your input with the as.numeric
function.
You can also compute the square root of a complex number or complex vector.
sqrt(8i)
2+2i
However, if you try to calculate the square root of a negative number the function will return a NaN
and a warning will arise indicating âNaNs producedâ.
sqrt(-1)
NaN
Warning message:
In sqrt(-1) : NaNs produced
In order to solve this issue you can use the abs
function to compute the absolute value of the input value or values and then compute the square root of the positive numbers.
sqrt(abs(-1))
1
Note that it is not possible to calculate the square root of a factor. If you try to do so you will get an error with the message âError in Math.factor(x) : âsqrtâ not meaningful for factorsâ.
x <- factor(c(3, 1, 6))
sqrt(x)
Error in Math.factor(x) : âsqrtâ not meaningful for factors
In order to solve this error you will need to transform you factor to numeric with the following line of code and then compute the square root of the numbers:
x <- factor(c(3, 1, 6))
x <- as.numeric(as.character(x))
sqrt(x)
1.732051 1.000000 2.449490
Plotting the square root in R
You can plot the square root for positive numbers using the following line of code. In this example we are plotting the square roots from 1 to 100.
plot(sqrt, 0, 100, col = 4, main = "sqrt(x)")
Nth root
The square root of a number is the number raised to the nth power. For instance, the square root of 8 is \(\sqrt{8} = 8 ^ {1/2} = 2.828427\).
# Square root of 8
8 ^ (1/2)
sqrt(8) # Equivalent
If you want to compute the cube root of a number you just need to raise the value to the 1/3 power, as shown in the example below.
# Cube power of 8
8 ^ (1/3)
2
The following function can be used to compute the cube root of any numeric value or vector. Below is a visual representation of the cube root from 0 to 100.
# Cube root function
cube_root <- function(x) {
return(x ^ (1/3))
}
plot(cube_root, 0, 100, col = 4, main = "Cubic root")
If you need to calculate the nth root of any number you can calculate the nth power by hand or create a function like the following to specify the input value and the desired power.
# Nth root function
nth_root <- function(x, nth) {
# x: input value
# nth: numeric value indicating the power (1/nth)
return(x ^ (1/nth))
}
# Example
nth_root(8, nth = 4) # 1.681793