Suspend the execution of an R code for a time interval
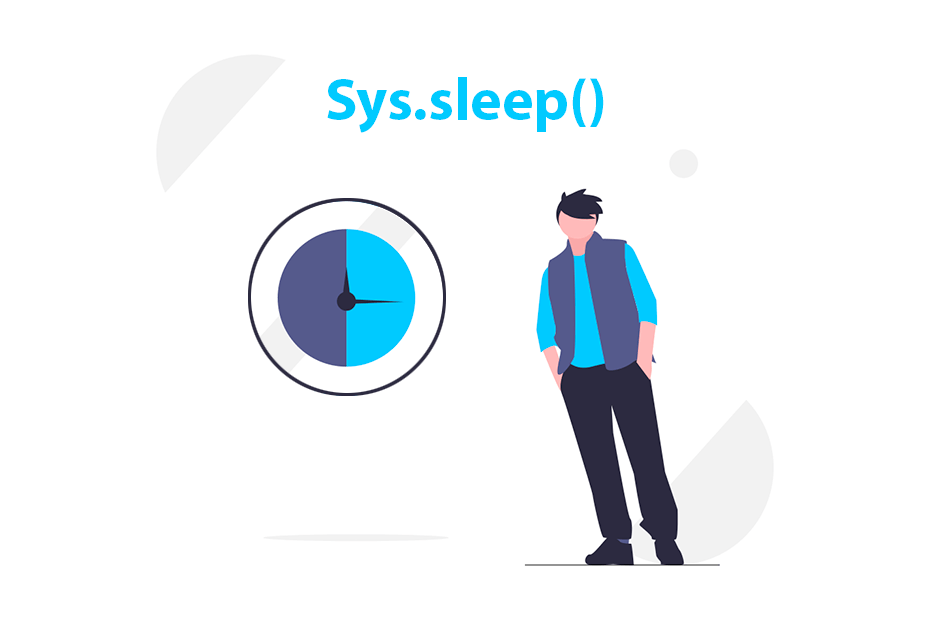
The Sys.sleep
function allows to stop the execution of an R process for a time interval, in seconds. This is very useful when you run a for loop and you need to slow down the speed of the loop.
Syntax and use cases
The function takes a positive number as input which will be the number of seconds to suspend the execution of the code.
Sys.sleep(time)
This function has several use cases. Some of them are:
- When you are scraping a website or downloading files and you don’t want to overload the server.
- To delay the execution of some code.
- When you want to retry some code that failed after some time (e.g. a server is down and you need to wait some time until is up).
- When you are making API calls to a limited API (e.g. it admits X calls per hour).
Using Sys.sleep
inside a for loop
Consider the following for loop that prints the execution time of each iteration:
for(i in 1:5) {
# Start time
start <- Sys.time()
# Code
# End time
end <- Sys.time()
# Time difference
time <- end - start
print(time)
}
Time difference of 2.861023e-06 secs
Time difference of 1.907349e-06 secs
Time difference of 1.907349e-06 secs
Time difference of 3.099442e-06 secs
Time difference of 9.536743e-07 secs
Each iteration takes almost no time to complete. However, if you want to stop the execution one second each iteration you can use Sys.sleep(1)
.
for(i in 1:5) {
# Start time
start <- Sys.time()
Sys.sleep(1)
# End time
end <- Sys.time()
# Time difference
time <- end - start
print(time)
}
Time difference of 1.003335 secs
Time difference of 1.012604 secs
Time difference of 1.014571 secs
Time difference of 1.028076 secs
Time difference of 1.027852 secs
Now each iteration takes around 1 more second to complete.
Using Sys.sleep
inside sapply
You can also use the Sys.sleep
function inside a sapply or a lapply function. In the following example we are pausing the execution 5 seconds between iterations.
sapply(1:5, function(i) {
Sys.sleep(5)
return(i)
})
1 2 3 4 5