Warning and error messages in R
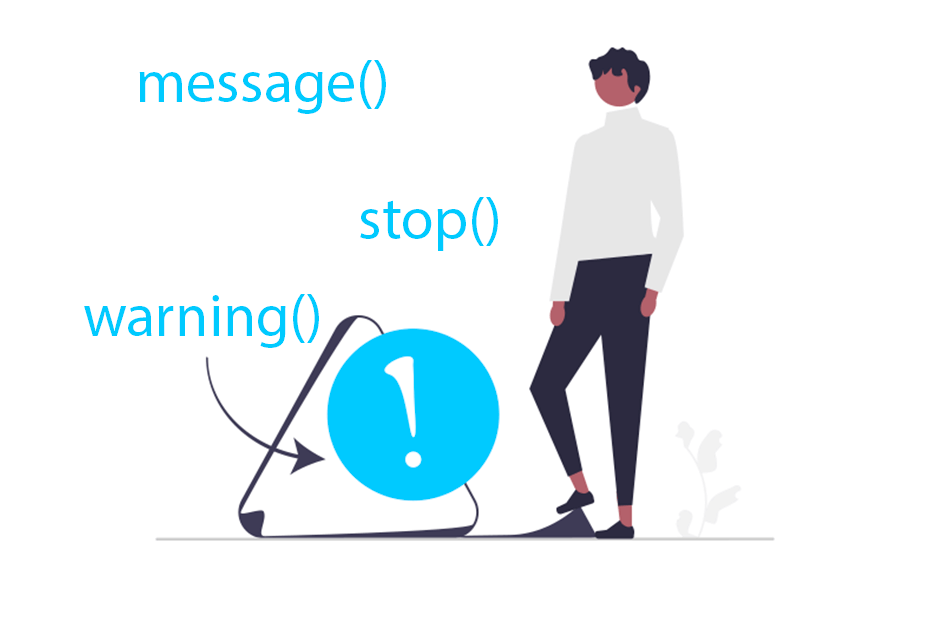
When using R, specially when creating functions, it is possible to add simple messages, warning messages or error messages to inform the user about something critical or non-critical with the message
, warning
and stop
functions. In this tutorial you will learn to create these messages and also to list or suppress these messages if possible.
Messages created with these functions will appear in the R console in orange.
Diagnostic messages with message()
The message
function prints a custom message on the console to inform the user about something, but not critical. Consider that you are writing a function and you want to inform the user to patiently wait until finish, so you add some messages that will be shown to the user, as in the example below:
# Sample function
test_fun <- function(x) {
message("The function is processing...please wait...")
# Main code
Sys.sleep(3)
message("Done")
}
test_fun()
The function is processing...please wait...
Done
If you are building a package and you want a startup message you can use the packageStartupMessage
function instead.
The function provides an argument named appendLF
which defaults to TRUE
to append a new line or not. If you set this argument to FALSE
the messages will be added to the same line.
# Sample function
test_fun <- function(x) {
message("The function is processing...please wait...", appendLF = FALSE)
# Main code
Sys.sleep(3)
message("Done", appendLF = FALSE)
}
test_fun()
The function is processing...please wait...Done
Suppress messages
If a function displays messages and you don’t want them to appear on your console you can wrap the function that produces those messages inside suppressMessages
to remove them.
# Sample function
test_fun <- function(x) {
message("The function is processing...please wait..."
# Main code
Sys.sleep(3)
message("Done")
}
suppressMessages(test_fun())
Note that you can also write more lines of code inside suppressMessages
if you use curly braces as shown in the example below.
# Sample function
test_fun <- function(x) {
message("The function is processing...please wait..."
# Main code
Sys.sleep(3)
message("Done")
}
suppressMessages({
# Code
test_fun()
test_fun()
})
In order to suppress package startup messages use the suppressPackageStartupMessages
function instead, e.g. suppressPackageStartupMessages(library(dplyr))
Warning messages with warning()
The warning
function is designed to inform the user about a potential issues on the code. In the following example we are showing a warning message if the input is not numeric.
# Sample function
test_warning <- function(x) {
if(!is.numeric(x)) {
warning("This is a warning message because x is not numeric")
}
return(x)
}
test_warning("15")
15
Warning message:
In test_warning(15) : This is a warning message because x is not numeric
List last warning messages
The warnings
function prints the last warning messages that appeared on console provided by the last.warning
variable.
test_warning("15")
# Show last warnings
warnings()
Warning message:
In test_warning("15") : This is a warning message because x is not numeric
Suppress warning messages
It is possible to ignore or suppress the warning message for a function with the suppressWarnings
function.
# Suppress warnings of a function
suppressWarnings(test_warning("15"))
# Suppress warnings for several lines of code
suppressWarnings({
test_warning("15")
test_warning("15")
})
An alternative to suppress warning message is to set warn = -1
inside options
, as shown in the example below. Recall to go back to the default setting (warn = 0
) to display warnings again.
# Ignore warnings
options(warn = -1)
test_warning("20")
# Back to default
options(warn = 0)
Error messages with stop()
The stop
function stops the execution of the code and throws the desired error message. This function is designed to stop the code when a critical error happens and to inform the user about it.
# Sample function
test_stop <- function(x) {
if(!is.numeric(x)) {
stop("'x' must be numeric")
} else {
x <- x * 2
}
return(x)
}
test_stop("12")
Error in test_stop("12") : 'x' must be numeric
Get last error message
The geterrmessage
function provides the last error message available as a character string.
geterrmessage()
Error in test_stop("12") : 'x' must be numeric