max, min, pmax and pmin functions in R
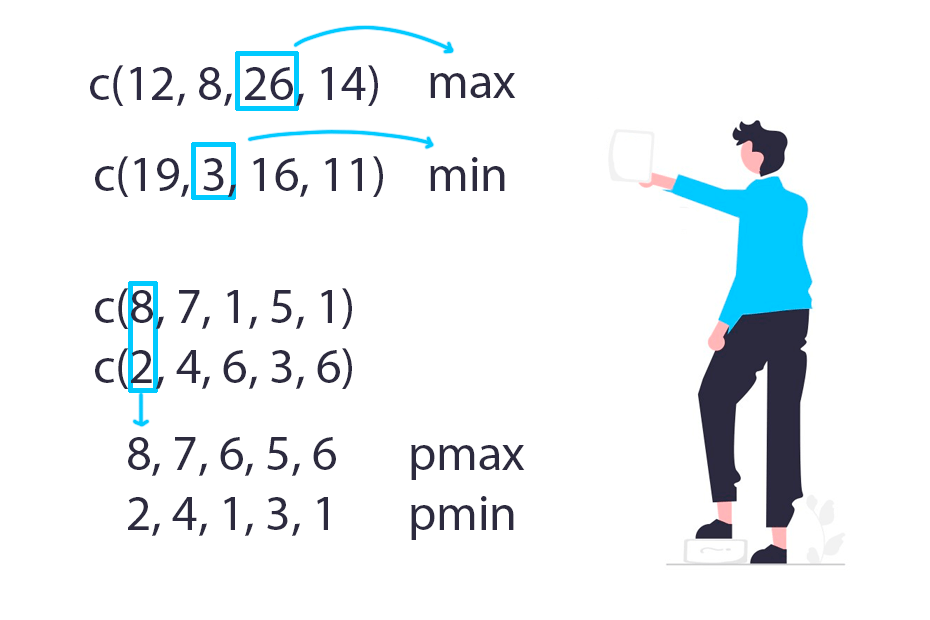
R provides several functions to look for maximum and minimum values. max
and min
will return the maximum and minimum value of a single vector, respectively and pmax
and pmin
will return the maximum and minimum values of several vectors in parallel.
max()
The max
function returns the maxima of the input vector. It returns only one value.
# Sample vector
x <- c(45, 12, 12, 15, 61, 56)
# Which is the maximum value?
max(x)
61
However, if the vector contains a missing value (NA
), the function will return an NA
.
# Sample vector
x <- c(45, 12, 12, NA, 15, 61, 56)
max(x)
NA
If you want to avoid this, you can set the na.rm
argument of the function as TRUE
, so missing values will be removed before getting the maximum value.
# Sample vector
x <- c(45, 12, 12, NA, 15, 61, 56)
max(x, na.rm = TRUE)
61
max(c(Inf, NA))
is NA
, despite the maximum possible value of NA
is Inf
.
min()
The min
function is the opposite of max
, as it will return the minimum value of the input vector. This function works the same way as the other function and also provides an argument named na.rm
which can be set to TRUE
to remove missing values, if any.
# Sample vector
x <- c(45, 12, 12, 15, 61, 56)
# Which is the minimum value?
min(x)
12
pmax()
The pmax
function is similar to max
but instead of a vector it can take several and it will return the maximum value between the values for each index of the vectors. For instance, in the following example, the first value returned is 52 because is the maxima between 45, 15 and 52, the second value returned is 35 because is the maxima between 12, 35 and 12, and so on.
# Sample vectors
x1 <- c(45, 12, 12, 15, 61, 56)
x2 <- c(15, 35, 81, 23, 45, 24)
x3 <- c(52, 12, 41, 35, 17, 16)
pmax(x1, x2, x3)
52 35 81 35 61 56
If the length of the vectors is different the function will return a vector of the length of the largest vector and the smallest vectors will be recycled. In the following example this implies that in the fifth element, the function will look for the maximum value between 61, 15 (recycled, first value of x1
) and 17 and in the last element the function will compare 56, 35 (recycled, second value of x2
) and 52 (recycled, first value of x3
).
# Sample vectors
x1 <- c(45, 12, 12, 15, 61, 56)
x2 <- c(15, 35, 81, 23)
x3 <- c(52, 12, 41, 35, 17)
pmax(x1, x2, x3)
52 35 81 35 61 56
Warning message:
In pmax(x1, x2, x3) : an argument will be fractionally recycled
Note that a warning message will arise if the length of the smallest vectors are not multiples of the largest vector.
pmin()
The pmin
function is the same as pmax
but it will return the minimum values element-wise for each index of the input vectors. In the example below the first value is 15 because is the minima between 45, 15 and 52, the second value is 12 because is the minima between 12, 35 and 12 and so on.
# Sample vectors
x1 <- c(45, 12, 12, 15, 61, 56)
x2 <- c(15, 35, 81, 23, 45, 24)
x3 <- c(52, 12, 41, 35, 17, 16)
pmin(x1, x2, x3)
15 12 12 15 17 16
This function will behave the same as pmax
if the lengths of the input vectors are different.