Convert objects to numeric with as.numeric()
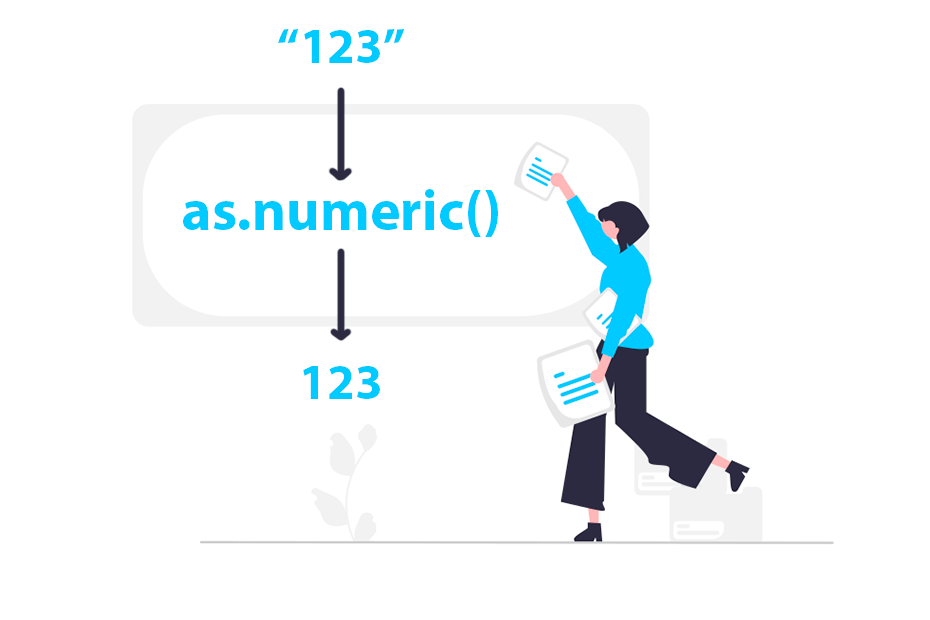
The as.numeric
function is a basic R function that converts R objects to numeric. In this tutorial you will learn how to coerce to numeric and how to check if an object is numeric or not.
Syntax and arguments
The syntax of the as.numeric
function is the following:
as.numeric(x, ...)
The function takes an object (x
) as argument, being:
- x: object to be coerced to numeric.
- âŠ: additional arguments if needed.
Usage
Character to numeric
Consider that you have a character vector containing numbers:
x <- c("19", "28", "26")
x
"19" "28" "26"
In this scenario, if you want to make mathematical operations you will get an error, like in the following example where we try to multiply the vector by two:
x <- c("19", "28", "26")
x <- x * 2
Error in x * 2 : non-numeric argument to binary operator
If you convert your vector to numeric now R will interpret the values as numbers, so you will be able to perform mathematical operations.
x <- c("19", "28", "26")
# Convert 'x' to numeric
x <- as.numeric(x)
x
19 28 26
Now you can multiply the values and get the desired result.
x <- x * 2
x
38 56 52
If the character vector contains non-numeric strings and you use the as.numeric
function that element will be converted to NA
and R will print âNAs introduced by coercionâ as a warning.
x <- c("19", "28", "26", "A")
# Convert 'x' to numeric
x <- as.numeric(x)
x
Warning message:
NAs introduced by coercion
> x
[1] 19 28 26 NA
Factor to numeric
Consider the following factor for illustration purposes:
f <- factor(c("3", "14", "15", "93"))
f
3 14 15 93
Levels: 14 15 3 93
Note that the levels of the factor doesnât have the same order as the input character vector.
If you want to convert the factor to numeric you will need to use the as.numeric
but also the as.character
function as follows:
f <- factor(c("3", "14", "15", "93"))
f <- as.numeric(as.character(f))
f
3 14 15 93
A more efficient alternative for long vectors is to use the following code:
f <- factor(c("3", "14", "15", "93"))
f <- as.numeric(levels(f))[f]
f
If you want to convert a factor to the original vector and with the same order never use as.numeric(f)
, as it wonât return the desired numeric vector. Learn more about factors.
Boolean to numeric
Consider that you have a boolean vector like the following:
b <- c(TRUE, FALSE, TRUE, TRUE, FALSE)
b
TRUE FALSE TRUE TRUE FALSE
You can convert the vector to numeric with the as.numeric
function which will convert TRUE
to 1 and FALSE
to 0.
b <- c(TRUE, FALSE, TRUE, TRUE, FALSE)
b <- as.numeric(b)
b
1 0 1 1 0
Check if an object is numeric with is.numeric
The is.numeric
function is useful to check if the data type of you object is numeric or not. If so, it will return TRUE
and FALSE
if not.
x <- c("19", "28", "26", "A")
# Check if x is numeric
is.numeric(x) # FALSE
# Convert 'x' to numeric
x <- as.numeric(x)
# Check again
is.numeric(x) # TRUE